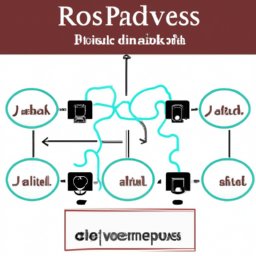
7.1. Working with routes in ExpressJS: Creating basic routes
Página 47
One of the most essential aspects when creating APIs in NodeJS is handling routes with ExpressJS. ExpressJS is a framework for NodeJS that provides a number of robust features for building web and mobile applications. It simplifies the process of creating routes for your application, making it easier to manage and maintain. In this chapter, we'll explore how to work with routes in ExpressJS, creating basic routes.
To begin with, we need to install ExpressJS. If you already have NodeJS installed, you can install ExpressJS using the following command in the terminal: npm install express
. After installation, we can start creating our first Express application. First, create a new file called 'app.js' and add the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!')
});
app.listen(port, () => {
console.log(`App listening at http://localhost:${port}`)
});
In this code, we first import the express module and create a new express application. Next, we define the port on which our application will be listening. Then we create a GET route to the homepage ('/') of our application. This route will respond with 'Hello World!' whenever someone lands on the homepage. Finally, we tell our application to start listening on the specified port.
To start the server, you can use the command node app.js
in the terminal. If you open a browser and go to 'http://localhost:3000', you will see the message 'Hello World!'.
This is the basics of how to create a route with ExpressJS. However, in most cases you will want to create multiple routes to different parts of your application. Let's see how to do this.
app.get('/about', (req, res) => {
res.send('About page')
});
app.get('/contact', (req, res) => {
res.send('Contact page')
});
Here we create two new routes, '/about' and '/contact'. Now, if you go to 'http://localhost:3000/about', you'll see the message 'About page', and if you go to 'http://localhost:3000/contact', you'll see the message 'Contact page'.
ExpressJS also allows you to create dynamic routes, that is, routes that can change depending on the data passed to them. This is done using route parameters. For example:
app.get('/users/:userId', (req, res) => {
res.send(`User ID is: ${req.params.userId}`)
});
In this example, ':userId' is a route parameter. You can access the value of this parameter using req.params.userId
. Now if you go to 'http://localhost:3000/users/123' you will see the message 'User ID is: 123'.
In addition to GET routes, ExpressJS also supports other HTTP methods such as POST, PUT, and DELETE. Here are some examples of how to create these routes:
app.post('/users', (req, res) => {
// Code to create a new user
res.send('User created')
});
app.put('/users/:userId', (req, res) => {
// Code to update a user
res.send('User updated')
});
app.delete('/users/:userId', (req, res) => {
// Code to delete a user
res.send('User deleted')
});
In each of these routes, you can add the necessary code to create, update, or delete a user, respectively.
In short, ExpressJS makes managing routes in your NodeJS application simple and intuitive. With it, you can create static and dynamic routes, support multiple HTTP methods, and organize your application neatly and efficiently. Once you've mastered routes in ExpressJS, you'll be well equipped to build robust, scalable APIs in NodeJS.
Now answer the exercise about the content:
What does ExpressJS allow you to create regarding routes in a NodeJS application?
You are right! Congratulations, now go to the next page
You missed! Try again.
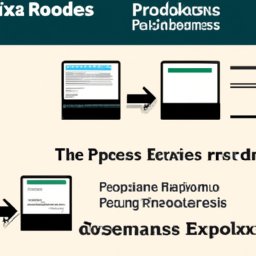
Next page of the Free Ebook: