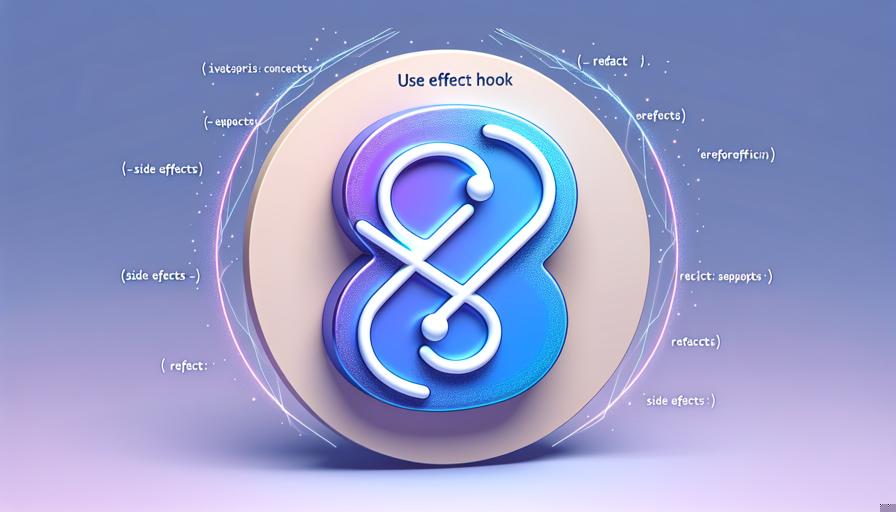
16. useEffect Hook: Performing Side Effects in React
Page 60 | Listen in audio
The useEffect
Hook is one of the most powerful and commonly used Hooks in React. It allows you to perform side effects in function components, which was previously only possible in class components using lifecycle methods such as componentDidMount
, componentDidUpdate
, and componentWillUnmount
. Understanding and using useEffect
effectively can significantly enhance your ability to build dynamic and efficient React applications.
At its core, useEffect
accepts two arguments: a function (often referred to as the effect function) and an optional dependencies array. The effect function contains the code that performs the side effect, while the dependencies array determines when the effect should be re-run. This design helps in optimizing performance by preventing unnecessary re-renders.
Basic Usage
To illustrate the basic usage of useEffect
, consider a simple example where we want to log a message to the console every time a component mounts:
import React, { useEffect } from 'react';
function ExampleComponent() {
useEffect(() => {
console.log('Component mounted');
}, []);
return <div>Check the console log!</div>;
}
In this example, the effect function logs a message to the console. The empty dependencies array []
ensures that this effect runs only once when the component mounts, similar to the behavior of componentDidMount
in class components.
Effect with Dependencies
The true power of useEffect
comes into play when you specify dependencies. When you pass variables in the dependencies array, the effect will only re-run if any of those variables change:
import React, { useState, useEffect } from 'react';
function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log(`Count has changed to ${count}`);
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
Here, the effect logs the count value to the console whenever it changes. This is because count
is included in the dependencies array. If you omit the dependencies array, the effect will run after every render, which can lead to performance issues if not handled carefully.
Cleaning Up Effects
Some effects require cleanup to prevent memory leaks or unwanted behavior. For instance, if you set up a subscription or a timer, you should clean it up when the component is unmounted or before the effect runs again. useEffect
allows you to return a cleanup function from the effect function:
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(prevSeconds => prevSeconds + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
return <div>Seconds: {seconds}</div>;
}
In this example, we use setInterval
to update the seconds every second. The cleanup function returned by the effect clears the interval, ensuring that it stops when the component unmounts or before the effect is re-run.
Multiple Effects
It's common to have multiple side effects in a single component. You can call useEffect
multiple times to handle different effects separately. This approach is beneficial for organizing code based on different concerns:
import React, { useState, useEffect } from 'react';
function UserProfile({ userId }) {
const [userData, setUserData] = useState(null);
const [isOnline, setIsOnline] = useState(false);
useEffect(() => {
// Fetch user data when userId changes
fetch(`/api/user/${userId}`)
.then(response => response.json())
.then(data => setUserData(data));
}, [userId]);
useEffect(() => {
// Set up a WebSocket connection to track online status
const connection = new WebSocket(`wss://example.com/user/${userId}`);
connection.onmessage = event => setIsOnline(event.data === 'online');
return () => connection.close();
}, [userId]);
return (
<div>
<h1>User Profile</h1>
{userData ? (
<div>Name: {userData.name}</div>
) : (
<p>Loading...</p>
)}
<p>Status: {isOnline ? 'Online' : 'Offline'}</p>
</div>
);
}
In this example, we use two separate effects: one for fetching user data and another for managing the online status. Each effect is concerned with a specific aspect of the component's behavior, making the code more modular and easier to maintain.
Common Pitfalls
While useEffect
is a powerful tool, it's essential to be aware of common pitfalls to avoid unintended behavior:
- Missing Dependencies: Failing to include all necessary dependencies in the dependencies array can lead to stale or incorrect data. Always ensure that any value used inside the effect is also listed in the dependencies array.
- Overuse: Using too many effects or effects that run too frequently can lead to performance issues. Consider whether an effect is necessary or if it can be optimized.
- Side Effects in Render: Avoid performing side effects directly in the render phase. Instead, use
useEffect
to ensure that side effects are executed at the appropriate time.
Conclusion
The useEffect
Hook is a fundamental part of building React applications, allowing you to perform side effects in function components. By understanding how to effectively use useEffect
, manage dependencies, and clean up effects, you can create more efficient and reliable React applications. As you become more comfortable with useEffect
, you'll find it an invaluable tool in your React development toolkit.
Now answer the exercise about the content:
What is the primary purpose of the `useEffect` Hook in React function components?
You are right! Congratulations, now go to the next page
You missed! Try again.
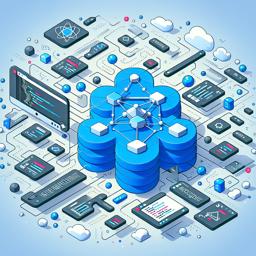
Next page of the Free Ebook: