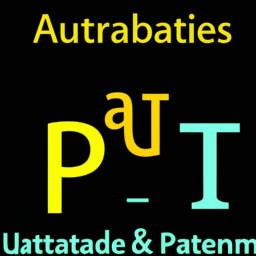
9. Unit Tests in Python
Página 37
Unit testing is an essential part of software development, and Python offers a number of powerful tools to facilitate this practice. In this section, we'll explore how unit testing is performed in Python, with a particular focus on using Lambda and API Gateway for backend development.
Unit testing in Python is generally performed using the unittest module, which is part of the standard library. This module provides a framework for creating and running tests, and includes a series of assertion functions that can be used to verify that code is behaving as expected.
Creating a unit test in Python involves creating a class that inherits from unittest.TestCase and then defining one or more test methods in that class. Each test method must begin with the word 'test' and must use the assertion functions provided by the unittest module to verify the behavior of the code.
For example, suppose we have a function that adds two numbers together. We could create a unit test for this function as follows:
import unittest def add(a, b): return a + b class TestAdd(unittest.TestCase): def test_add(self): self.assertEqual(add(1, 2), 3)
In this example, the test_add method checks whether the add function returns the expected value when called with arguments 1 and 2. If the add function does not return 3 when called with these arguments, the test fails.
In addition to the unittest module, Python also supports a number of other testing tools, including pytest and nose. These tools provide additional functionality, such as the ability to write parameterized tests and the ability to use fixtures to configure and clear test state.
When working with Lambda and API Gateway, unit tests can be a little more complex, as it is necessary to verify not only the behavior of the code, but also the interaction between the code and the AWS infrastructure.
For example, if you are using Lambda to run a function whenever a request is made to a certain API Gateway endpoint, you need to verify that the Lambda function is being called correctly and that it is returning the expected response. This may involve creating mockups for the input event and Lambda context, and then verifying that the Lambda function correctly handles those mockups.
In addition, you will also need to verify that your API Gateway configuration is correct. This may involve checking things like your routing settings, security settings, and Lambda integration settings.
In summary, unit testing is an essential practice in software development and is especially important when working with complex infrastructures like Lambda and API Gateway. Python offers a number of powerful tools to make writing and running unit tests easier, and it's important to make good use of these tools to ensure your code is robust and reliable.
In the next section, we'll explore more about how these tools can be used in conjunction with Lambda and API Gateway to develop and test efficient and reliable backend applications.
Now answer the exercise about the content:
What is the main function of the unittest module in Python and how is a unit test created?
You are right! Congratulations, now go to the next page
You missed! Try again.
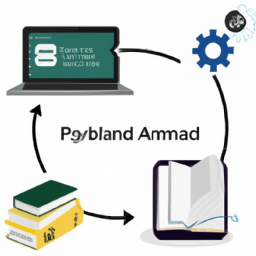
Next page of the Free Ebook: