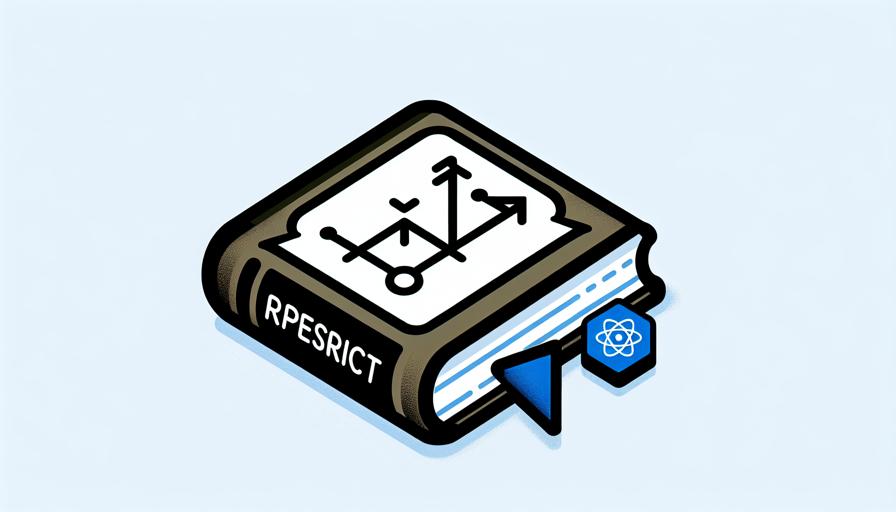
41. Understanding and Using TypeScript with React
Page 85 | Listen in audio
TypeScript is a powerful tool that enhances the development experience in JavaScript applications, and its integration with React has been a game-changer for many developers. Understanding and using TypeScript with React can significantly improve the robustness and maintainability of your codebase. This section will guide you through the essentials of using TypeScript in a React environment, helping you to harness its full potential.
What is TypeScript?
TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. It offers static typing, which can help catch errors early in the development process. By adding types to JavaScript, TypeScript provides a more structured and predictable development experience, making it easier to manage large codebases.
Why Use TypeScript with React?
- Type Safety: TypeScript allows you to define types for your variables, function parameters, and return values, reducing the likelihood of runtime errors.
- Better Tooling: With TypeScript, you get improved autocompletion, navigation, and refactoring in your IDE, which can significantly enhance productivity.
- Documentation: Types can serve as a form of documentation for your code, making it easier for others (and yourself) to understand the intended use of components and functions.
- Maintaining Large Codebases: TypeScript’s static typing can help manage and maintain large React applications by making it easier to refactor code safely.
Setting Up TypeScript in a React Project
To start using TypeScript in a React project, you can either create a new project with TypeScript or add TypeScript to an existing project.
Creating a New React Project with TypeScript
You can create a new React project with TypeScript using Create React App by running the following command:
npx create-react-app my-app --template typescript
This command sets up a new React project with TypeScript configured out of the box.
Adding TypeScript to an Existing React Project
If you have an existing React project and want to add TypeScript, you can do so by installing the necessary dependencies:
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
Next, rename your .js
files to .tsx
, which is the file extension for TypeScript files that contain JSX. You’ll also need to create a tsconfig.json
file in the root of your project to configure TypeScript.
Basic TypeScript Concepts for React
Understanding TypeScript’s basic concepts is crucial for effectively using it with React. Here are some key concepts:
Types and Interfaces
Types and interfaces allow you to define the shape of objects and functions. Here’s an example of using an interface to define props for a React component:
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => (
<button onClick={onClick}>{label}</button>
);
In this example, the ButtonProps
interface defines the types for the label
and onClick
props.
Union and Intersection Types
Union types allow a variable to hold more than one type, while intersection types combine multiple types into one. Here’s an example:
type Status = 'success' | 'error' | 'loading';
interface User {
name: string;
age: number;
}
interface Admin {
admin: boolean;
}
type AdminUser = User & Admin;
In this case, Status
is a union type, and AdminUser
is an intersection type.
Generics
Generics allow you to create reusable components that can work with any data type. Here’s an example of a generic component:
interface ListProps<T> {
items: T[];
renderItem: (item: T) => React.ReactNode;
}
const List = <T extends {}>({ items, renderItem }: ListProps<T>) => (
<ul>
{items.map((item, index) => (
<li key={index}>{renderItem(item)}</li>
))}
</ul>
);
This List
component can render a list of any type of items, as specified by the generic type T
.
Advanced TypeScript Features for React
Once you’re comfortable with the basics, you can explore more advanced TypeScript features to further enhance your React applications.
Type Inference
TypeScript can often infer types based on the values you assign to variables, making your code more concise. For example:
let message = 'Hello, world!'; // TypeScript infers the type as 'string'
Discriminated Unions
Discriminated unions are useful for handling different types of data in a type-safe way. Here’s an example:
interface LoadingState {
state: 'loading';
}
interface SuccessState {
state: 'success';
data: string;
}
interface ErrorState {
state: 'error';
error: string;
}
type RequestState = LoadingState | SuccessState | ErrorState;
function handleRequest(state: RequestState) {
switch (state.state) {
case 'loading':
console.log('Loading...');
break;
case 'success':
console.log('Data:', state.data);
break;
case 'error':
console.log('Error:', state.error);
break;
}
}
This pattern ensures that you handle all possible states of a request.
Utility Types
TypeScript provides several utility types to facilitate common type transformations. Some useful utility types include:
Partial<T>
: Makes all properties inT
optional.Required<T>
: Makes all properties inT
required.Readonly<T>
: Makes all properties inT
readonly.Pick<T, K>
: Creates a type by picking a set of propertiesK
fromT
.Omit<T, K>
: Creates a type by omitting a set of propertiesK
fromT
.
Integrating TypeScript with React Libraries
Many popular React libraries have TypeScript support, which makes integrating them into your TypeScript React projects seamless. Libraries like React Router, Redux, and Material-UI provide type definitions to ensure type safety and improve the development experience.
Conclusion
Incorporating TypeScript into your React projects can lead to more robust and maintainable code. By leveraging TypeScript’s powerful type system, you can catch errors early, improve your development workflow, and create more reliable applications. As you become more familiar with TypeScript, you’ll find that it becomes an invaluable tool in your React development toolkit.
Now answer the exercise about the content:
What is one of the main benefits of using TypeScript with React?
You are right! Congratulations, now go to the next page
You missed! Try again.
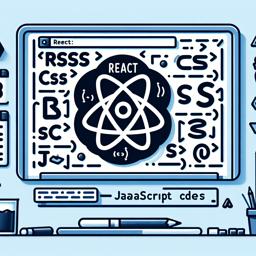
Next page of the Free Ebook: