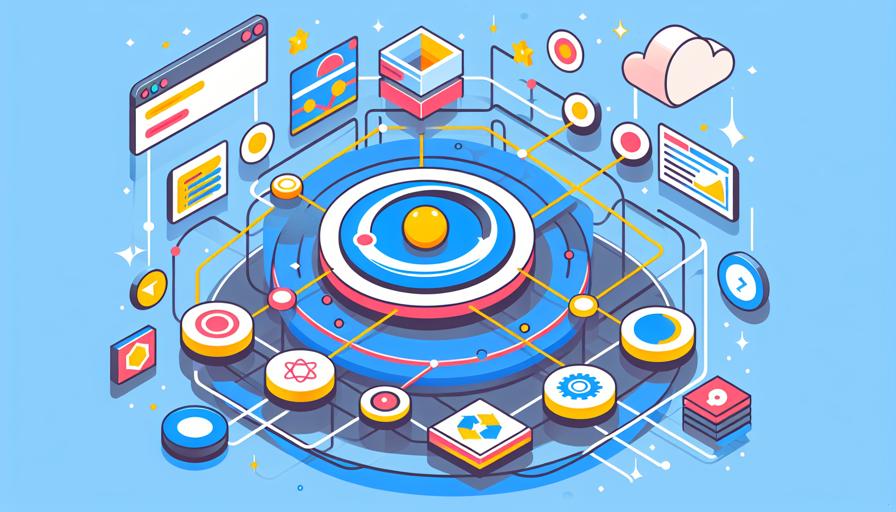
9.15. State: Managing State in React: State Management in Server-Side Rendering
Page 39 | Listen in audio
Managing state in React applications is a crucial aspect of building dynamic and interactive web applications. When it comes to server-side rendering (SSR), state management takes on additional complexity. SSR involves rendering your React components on the server and sending the HTML to the client, which can improve performance and SEO. However, managing state in this context requires careful consideration to ensure that your application remains efficient and consistent.
At its core, state management in SSR involves synchronizing the state between the server and the client. This synchronization is essential to ensure that the client-side application picks up where the server-side rendering left off, providing a seamless user experience. There are several strategies and patterns to manage state effectively in SSR, each with its own benefits and trade-offs.
Understanding the Basics of State in React
In React, state is an object that determines how a component renders and behaves. State can change over time, usually in response to user actions or network responses. React provides a built-in mechanism to manage state within components using the useState
hook or the setState
method in class components. However, when dealing with large applications, especially those utilizing SSR, local component state can become unwieldy.
For SSR, the initial state of your application is often determined on the server. This involves fetching data and preparing the state before the HTML is sent to the client. Once the HTML is delivered, the client-side React application takes over, and the state needs to be rehydrated to match the server-rendered content. This process ensures that there is no mismatch between what the user sees initially and what the client-side application renders.
Challenges of State Management in SSR
One of the primary challenges of state management in SSR is ensuring that the state is consistent across server and client. This involves several key considerations:
- Data Fetching: In SSR, data fetching often occurs on the server to populate the initial state. This can be complex, as it involves making network requests and handling asynchronous operations before rendering the HTML.
- State Hydration: After the server sends the HTML to the client, the client-side React application must "hydrate" the initial state. This means reusing the server-rendered HTML and attaching event listeners to make the application interactive.
- Performance: SSR can improve performance by reducing the time to first paint, but it can also introduce latency due to server-side data fetching. Balancing these factors is crucial for an optimal user experience.
- SEO: One of the main reasons for using SSR is to improve SEO by providing fully-rendered HTML to search engines. State management must ensure that the content is complete and accurate for indexing.
Strategies for State Management in SSR
Several strategies can be employed to manage state effectively in SSR applications:
1. Global State Management Libraries
Libraries like Redux or MobX can be used to manage global state in SSR applications. These libraries provide a centralized store for state, which can be accessed and modified from both the server and client. Using a global state management library allows you to:
- Initialize the state on the server before rendering.
- Serialize the state and send it to the client as part of the HTML payload.
- Rehydrate the state on the client to ensure consistency.
While this approach provides a robust solution for state management, it can introduce additional complexity and boilerplate code. Careful planning is required to ensure that state transitions are handled correctly across server and client.
2. Context API
The React Context API offers a simpler alternative to global state management libraries. It allows you to share state across components without passing props down manually. For SSR, you can:
- Create a context provider on the server to initialize state.
- Wrap your application with the context provider to make the state available to all components.
- Use the context consumer to access the state in your components.
While the Context API is less complex than libraries like Redux, it may not be suitable for very large applications with complex state logic.
3. Data Fetching Libraries
Libraries like React Query or SWR can be used to handle data fetching and caching in SSR applications. These libraries abstract the complexities of data fetching and provide hooks to manage asynchronous operations. For SSR, you can:
- Fetch data on the server and cache it.
- Send the cached data to the client as part of the initial HTML.
- Use hooks provided by the library to access and update the cached data on the client.
Data fetching libraries can simplify the process of handling asynchronous state and reduce the amount of boilerplate code required for SSR.
Implementing State Management in SSR
To implement state management in an SSR application, follow these general steps:
Step 1: Server-Side Data Fetching
On the server, fetch the necessary data to populate the initial state. This might involve calling APIs, querying databases, or performing other asynchronous operations. Once the data is fetched, initialize the state with this data.
Step 2: Render HTML with Initial State
Use the fetched data to render the HTML on the server. This HTML will be sent to the client as the initial view of the application. Ensure that the state is serialized and included in the HTML payload, often as a JSON object in a script tag.
Step 3: Client-Side State Hydration
On the client, rehydrate the application by using the initial state provided by the server. This involves parsing the JSON object and using it to initialize the client-side state. The client-side React application will then take over, making the application interactive.
Step 4: Synchronize State Changes
Ensure that any state changes on the client are synchronized with the server if necessary. This might involve sending updates back to the server or fetching new data as needed. Use the chosen state management strategy to handle these updates efficiently.
Conclusion
Managing state in server-side rendered React applications is a complex but rewarding endeavor. By carefully considering the challenges and employing appropriate strategies, you can build applications that are performant, SEO-friendly, and provide a seamless user experience. Whether you choose a global state management library, the Context API, or a data fetching library, the goal is to ensure consistency and efficiency across server and client.
As you continue to develop React applications with SSR, keep exploring and experimenting with different state management patterns to find the best fit for your specific needs. With practice, you'll be able to leverage the full power of SSR while maintaining robust and maintainable state management.
Now answer the exercise about the content:
What is one of the main reasons for using server-side rendering (SSR) in React applications?
You are right! Congratulations, now go to the next page
You missed! Try again.
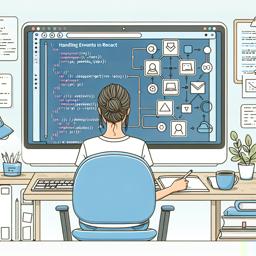
Next page of the Free Ebook: