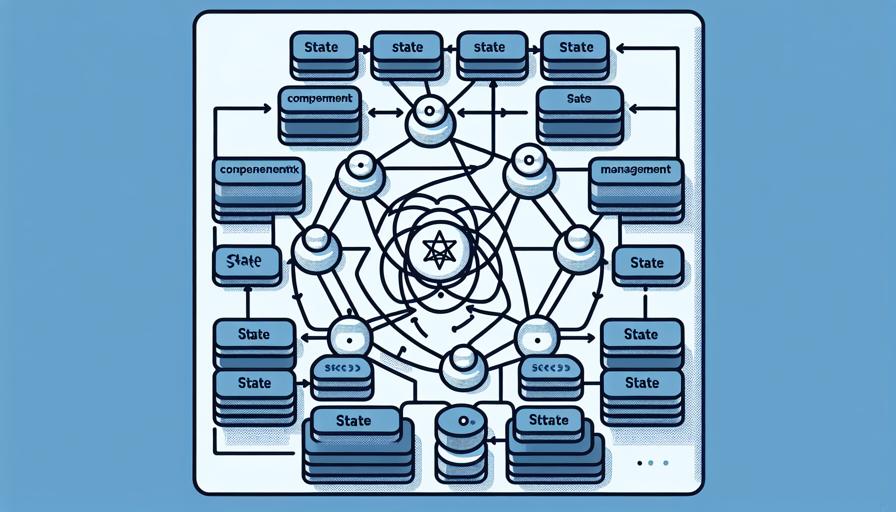
9.11. State: Managing State in React: State Management in Large Applications
Page 35 | Listen in audio
Managing state in React is a fundamental aspect of building dynamic and interactive applications. As applications grow in complexity, managing state efficiently becomes crucial to ensure maintainability, performance, and scalability. In this section, we'll explore how to manage state in large React applications, discussing various strategies and tools that can help streamline this process.
State management in React revolves around the concept of "state," which refers to the data that changes over time within a component. This state affects how the component renders and behaves. In smaller applications, managing state might be straightforward, but as the application scales, you need more robust solutions to handle state across multiple components and modules.
Understanding State in React
In React, state is typically managed within components using the useState
hook for functional components or the this.setState
method for class components. This local state is ideal for managing data that is specific to a component and doesn't need to be shared across other components. However, in large applications, relying solely on local state can lead to challenges, including:
- Prop Drilling: Passing state and functions through multiple component layers can become cumbersome and error-prone.
- State Duplication: Different components managing the same piece of state can lead to inconsistencies and bugs.
- Complex State Logic: Managing complex state logic within individual components can make them difficult to understand and maintain.
Centralized State Management
To address these challenges, centralized state management solutions like Redux, MobX, or the Context API can be used. These tools allow you to manage state in a single location and access it from any component in your application, reducing the need for prop drilling and state duplication.
Redux
Redux is a popular state management library that follows the principles of a unidirectional data flow and emphasizes immutability. It uses a single store to manage the entire application state, making it easy to debug and test. Redux also provides middleware support, allowing you to handle asynchronous actions and side effects efficiently.
Using Redux involves setting up actions, reducers, and a store. Actions describe what happened, reducers specify how the state changes in response to actions, and the store holds the entire application state. By connecting components to the Redux store, you can access and update state seamlessly.
MobX
MobX is another state management library that provides a more flexible and less boilerplate-heavy approach compared to Redux. It uses observable objects to track state changes and automatically update components that depend on that state. MobX is particularly useful for applications where you need to manage complex and mutable state.
With MobX, you define state as observable properties and use actions to modify that state. Components automatically react to changes in observable properties, making it easy to manage state without manually connecting components to a central store.
Context API
The Context API is a built-in feature of React that allows you to share state across components without prop drilling. It's suitable for managing global state that doesn't require the full capabilities of Redux or MobX. The Context API is often used for theming, authentication, and other application-wide settings.
To use the Context API, you create a context object using React.createContext()
and provide it to components via a context provider. Components can then consume the context using the useContext
hook or the Context.Consumer
component.
Choosing the Right State Management Solution
When deciding which state management solution to use, consider the following factors:
- Application Complexity: For small to medium-sized applications, the Context API may be sufficient. For larger applications with complex state logic, Redux or MobX might be more appropriate.
- Team Familiarity: Consider the team's familiarity with different state management libraries. Redux has a steeper learning curve but a large community and ecosystem, while MobX offers a more intuitive approach.
- Performance Requirements: Evaluate the performance implications of each solution. Redux's immutability can lead to performance optimizations, while MobX's reactivity can simplify state updates.
Best Practices for State Management
Regardless of the state management solution you choose, following best practices can help you maintain a clean and efficient codebase:
- Keep Components Stateless: Whenever possible, keep components stateless and derive their state from props or a central store. This makes components more reusable and easier to test.
- Normalize State: Normalize your state structure to avoid duplication and inconsistencies. Use libraries like
normalizr
to flatten nested data structures. - Use Selectors: Use selectors to derive data from the state. Selectors can help you encapsulate complex state logic and improve performance by memoizing results.
- Group Related State: Group related state into modules or slices to keep your state organized and maintainable. This approach is often referred to as "duck" patterns in Redux.
- Handle Side Effects: Use middleware like
redux-thunk
orredux-saga
to handle asynchronous actions and side effects. This keeps your action creators and reducers pure.
Conclusion
Managing state in large React applications requires careful consideration of the tools and strategies you use. Whether you opt for Redux, MobX, or the Context API, understanding the trade-offs and best practices associated with each approach is essential. By centralizing state management and following best practices, you can build scalable, maintainable, and performant React applications that meet the needs of your users and development team.
As you continue to develop your React skills, remember that state management is just one piece of the puzzle. Combining effective state management with other React patterns and practices will empower you to create robust and dynamic web applications.
Now answer the exercise about the content:
What is a primary reason for using centralized state management solutions like Redux or MobX in large React applications?
You are right! Congratulations, now go to the next page
You missed! Try again.
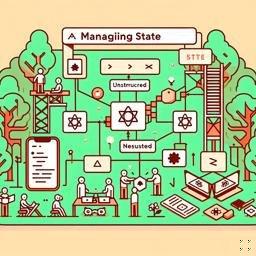
Next page of the Free Ebook: