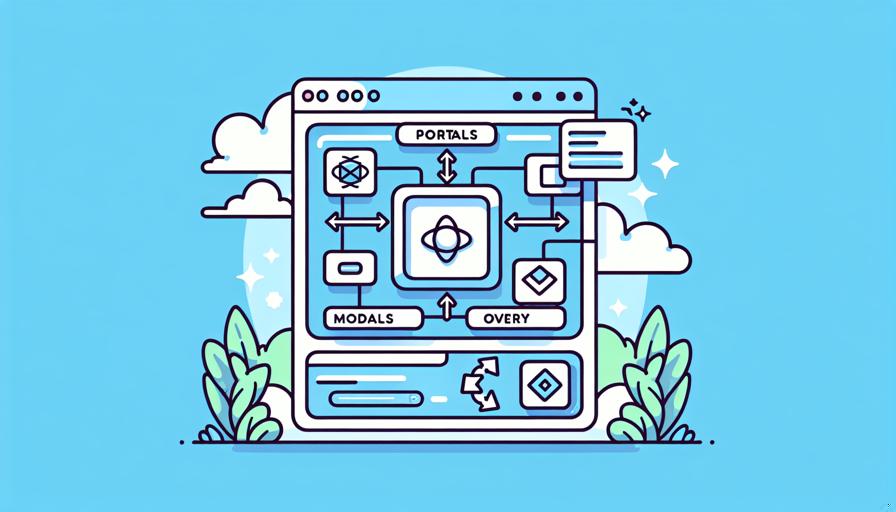
29. React Portals: Working with Modals and Overlays
Page 73 | Listen in audio
In the journey of mastering React, understanding how to manipulate the DOM effectively is crucial. React Portals offer a powerful way to render components outside the main DOM hierarchy of your application. This feature is particularly useful when dealing with UI elements like modals, tooltips, and overlays that require a higher z-index or need to escape certain styling constraints imposed by parent components.
React Portals provide a first-class way to render children into a DOM node that exists outside the DOM hierarchy of the parent component. This capability is essential when you need to break out of the parent component's styling or structure, such as when implementing a modal dialog that should overlay the entire screen.
Understanding React Portals
React Portals are created using the ReactDOM.createPortal
method. This method takes two arguments: the JSX or React component you want to render and the DOM node where you want it to be rendered. Here's a basic example:
const modalRoot = document.getElementById('modal-root');
function MyPortalComponent() {
return ReactDOM.createPortal(
<div>This is rendered using a portal!</div>,
modalRoot
);
}
In this example, modalRoot
is a DOM node outside the main app root. The component MyPortalComponent
will render its content into this node, effectively bypassing the usual parent-child DOM structure.
Implementing Modals with React Portals
Modals are a common use case for React Portals. They often need to overlay the entire screen and should not be constrained by the parent component's styling. Let's walk through building a simple modal using React Portals.
Step 1: Setting Up the HTML
First, ensure your HTML file has a dedicated div
for the portal. This is typically placed outside your main root
div:
<div id="root"></div>
<div id="modal-root"></div>
Step 2: Creating the Modal Component
Next, create a Modal component that uses ReactDOM.createPortal
to render its content:
import React from 'react';
import ReactDOM from 'react-dom';
const Modal = ({ isOpen, onClose, children }) => {
if (!isOpen) return null;
return ReactDOM.createPortal(
<div style={modalStyle}>
<div style={overlayStyle} onClick={onClose} />
<div style={modalContentStyle}>
<button onClick={onClose}>Close</button>
{children}
</div>
</div>,
document.getElementById('modal-root')
);
};
const modalStyle = {
position: 'fixed',
top: 0,
left: 0,
width: '100%',
height: '100%',
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
zIndex: 1000,
};
const overlayStyle = {
position: 'absolute',
top: 0,
left: 0,
width: '100%',
height: '100%',
backgroundColor: 'rgba(0, 0, 0, 0.5)',
};
const modalContentStyle = {
position: 'relative',
backgroundColor: '#fff',
padding: '20px',
borderRadius: '5px',
zIndex: 1001,
};
export default Modal;
In this component, the modal content is rendered into the modal-root
node. The modal is styled to cover the entire screen with a semi-transparent overlay and a centered content box.
Step 3: Using the Modal Component
Now, use the Modal component in your application:
import React, { useState } from 'react';
import Modal from './Modal';
function App() {
const [isModalOpen, setModalOpen] = useState(false);
return (
<div>
<h1>My React App</h1>
<button onClick={() => setModalOpen(true)}>Open Modal</button>
<Modal isOpen={isModalOpen} onClose={() => setModalOpen(false)}>
<h2>This is a modal!</h2>
<p>You can put any content here.</p>
</Modal>
</div>
);
}
export default App;
In this example, clicking the "Open Modal" button sets isModalOpen
to true
, which causes the Modal to render. Clicking the overlay or the "Close" button will set isModalOpen
to false
, hiding the modal.
Benefits of Using React Portals
React Portals offer several advantages when working with modals and overlays:
- Styling Independence: Portals allow components to escape the styling constraints of their parent components. This is particularly useful for modals that need to overlay the entire screen.
- Improved Accessibility: By rendering modals outside the main app structure, you can manage focus and keyboard interactions more effectively, enhancing accessibility.
- Separation of Concerns: Portals help maintain a clean separation between the modal's implementation and the rest of your application, making your code easier to manage and reason about.
Handling Events and Accessibility
When implementing modals, it's important to handle events and accessibility considerations effectively. Ensure that focus is managed correctly when the modal opens and closes. You can use libraries like focus-trap-react
to trap focus within the modal while it's open.
Additionally, make sure your modals are announced to screen readers by setting appropriate ARIA roles and attributes. For instance, you can set role="dialog"
and use aria-labelledby
and aria-describedby
attributes to provide context and descriptions for screen reader users.
Conclusion
React Portals are a powerful feature that can greatly enhance the flexibility and usability of your application's UI components. By allowing components to render outside their usual DOM hierarchy, they enable you to create dynamic and accessible modals, overlays, and other UI elements that break free from styling constraints.
As you continue to explore React, consider how Portals can be leveraged in your projects to solve complex UI challenges. With a solid understanding of this feature, you'll be well-equipped to build more versatile and maintainable React applications.
Now answer the exercise about the content:
What is one of the main advantages of using React Portals for modals and overlays?
You are right! Congratulations, now go to the next page
You missed! Try again.
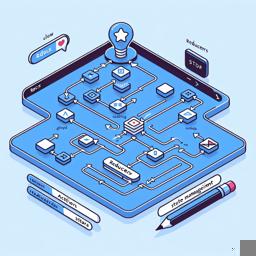
Next page of the Free Ebook: