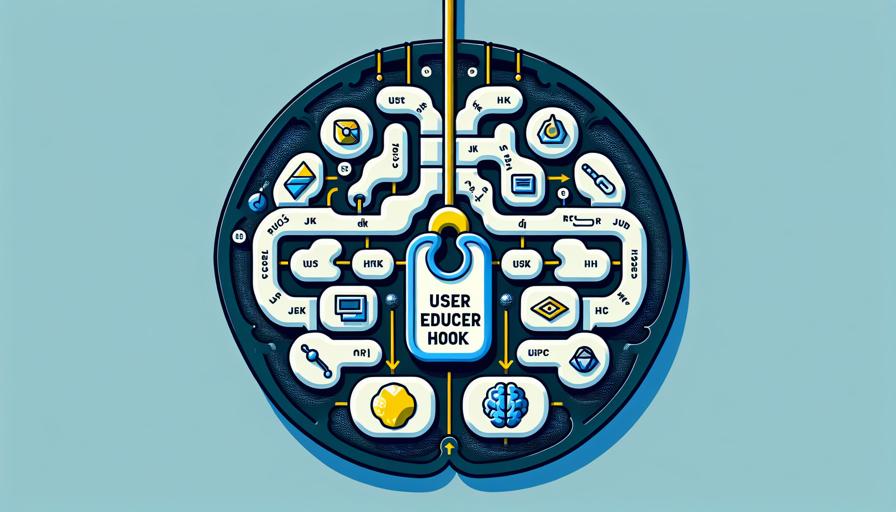
14.5. React Hooks: An Introduction: useReducer Hook: Managing Complex State Logic
Page 49 | Listen in audio
React Hooks have revolutionized the way developers build and manage state in functional components. Among the various hooks provided by React, useReducer
stands out as a powerful tool for managing complex state logic. It is particularly useful when the state logic involves multiple sub-values or when the next state depends on the previous one. In this section, we will delve into the useReducer
hook, exploring its capabilities and understanding how it can be effectively utilized to manage complex state logic in React applications.
At its core, useReducer
is a hook that allows you to manage state in a React component using a reducer function. This approach is reminiscent of Redux, a popular state management library, where state transitions are handled by reducers. The main advantage of using useReducer
is that it provides a more structured and predictable way to manage state transitions, especially when dealing with intricate state changes.
Understanding the Basics
The useReducer
hook requires two primary elements: a reducer function and an initial state. The reducer function is a pure function that takes the current state and an action as arguments, and returns a new state. The initial state is the state value before any actions have been dispatched.
const [state, dispatch] = useReducer(reducer, initialState);
In this setup, state
represents the current state, and dispatch
is a function that you call to update the state. The dispatch
function takes an action as its argument, which is then processed by the reducer function to produce a new state.
Defining a Reducer Function
The reducer function is central to the useReducer
hook. It takes the current state and an action object, and based on the action type, it returns a new state. Here is a simple example of a reducer function:
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
In this example, the state is an object with a single property, count
. The reducer function handles two action types: increment
and decrement
. Depending on the action type, it returns a new state with the updated count value.
Using useReducer
in a Component
To use the useReducer
hook in a React component, you first need to import it from React. Then, you define your reducer function and initial state, and call useReducer
with these parameters. Here is an example:
import React, { useReducer } from 'react';
function Counter() {
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
}
export default Counter;
In this example, the Counter
component uses the useReducer
hook to manage the count state. The component renders two buttons that, when clicked, dispatch actions to increment or decrement the count.
Managing Complex State Logic
While useReducer
is straightforward for simple state updates, its real power shines when managing complex state logic. Consider a scenario where you have a form with multiple fields, and you need to handle various actions like updating field values, resetting the form, and submitting the form. Instead of managing each field's state individually, you can use useReducer
to handle all these actions in a single, cohesive manner.
Example: Form State Management
Let's build a form component that uses useReducer
to manage its state:
import React, { useReducer } from 'react';
const initialState = {
username: '',
email: '',
password: '',
submitted: false,
};
function reducer(state, action) {
switch (action.type) {
case 'updateField':
return {
...state,
[action.field]: action.value,
};
case 'reset':
return initialState;
case 'submit':
return {
...state,
submitted: true,
};
default:
return state;
}
}
function Form() {
const [state, dispatch] = useReducer(reducer, initialState);
const handleChange = (e) => {
dispatch({
type: 'updateField',
field: e.target.name,
value: e.target.value,
});
};
const handleSubmit = (e) => {
e.preventDefault();
dispatch({ type: 'submit' });
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
name="username"
value={state.username}
onChange={handleChange}
placeholder="Username"
/>
<input
type="email"
name="email"
value={state.email}
onChange={handleChange}
placeholder="Email"
/>
<input
type="password"
name="password"
value={state.password}
onChange={handleChange}
placeholder="Password"
/>
<button type="submit">Submit</button>
<button type="button" onClick={() => dispatch({ type: 'reset' })}>Reset</button>
{state.submitted && <p>Form submitted!</p>}
</form>
);
}
export default Form;
In this example, the form component uses useReducer
to manage multiple fields and actions. The reducer function handles three types of actions: updating a field value, resetting the form to its initial state, and marking the form as submitted. This approach provides a clear and organized way to manage form state, making it easier to maintain and extend.
Benefits of Using useReducer
The useReducer
hook offers several benefits, especially when dealing with complex state logic:
- Predictable State Transitions: By centralizing state updates in a single reducer function,
useReducer
ensures that state transitions are predictable and easy to trace. - Structured State Management: The reducer function provides a structured approach to state management, making it easier to handle multiple actions and state changes.
- Improved Readability: By organizing state logic in a reducer function, your components become more readable, as the state management logic is separated from the component's rendering logic.
- Scalability: As your application grows,
useReducer
can help manage increasingly complex state logic without cluttering your component code.
When to Use useReducer
While useReducer
is a powerful tool, it is not always the best choice for every situation. Here are some guidelines on when to use useReducer
:
- Complex State Logic: If your component's state logic is complex, involving multiple sub-values or intricate updates,
useReducer
can provide a more organized and maintainable solution. - Dependent State Updates: When the next state depends on the previous state,
useReducer
can help ensure that state transitions are handled correctly. - Multiple State Changes: If you find yourself managing multiple related state changes,
useReducer
can help consolidate these changes into a single, cohesive logic.
In contrast, for simple state management tasks, such as toggling a boolean value or managing a single state variable, the useState
hook might be more appropriate due to its simplicity.
In conclusion, the useReducer
hook is a valuable addition to the React Hooks family, offering a structured and scalable approach to managing complex state logic. By understanding how to define reducer functions, manage actions, and utilize the useReducer
hook effectively, developers can build robust and maintainable React applications. Whether you're building a simple counter or a complex form, useReducer
provides the tools needed to manage state transitions with confidence and clarity.
Now answer the exercise about the content:
What is the primary advantage of using the `useReducer` hook in React for managing state transitions?
You are right! Congratulations, now go to the next page
You missed! Try again.
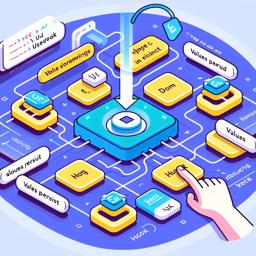
Next page of the Free Ebook: