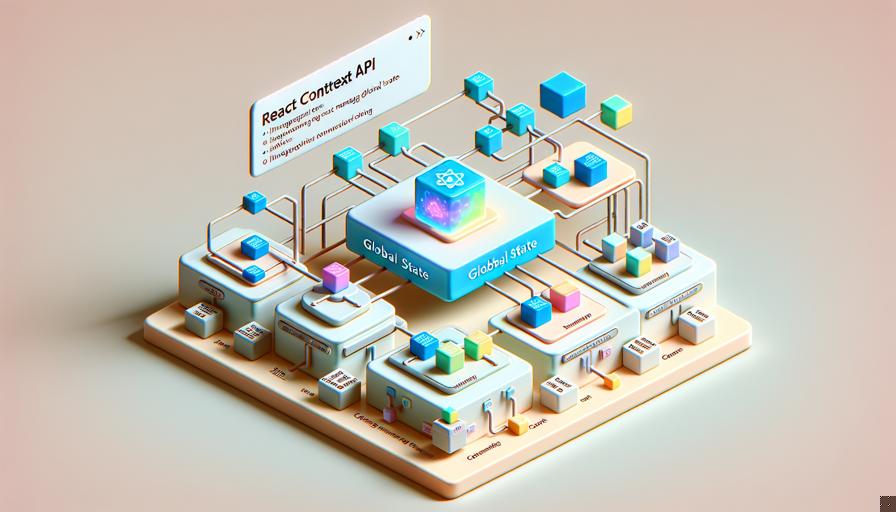
22. React Context API: Managing Global State
Page 66 | Listen in audio
In the world of React development, managing state is a crucial aspect of building efficient and scalable applications. As your application grows, passing state down through multiple layers of components can become cumbersome and error-prone. This is where the React Context API comes into play, offering a way to manage global state that can be accessed by any component in the tree without having to pass props down manually at every level.
The React Context API was introduced to solve the problem of prop drilling, where props are passed through multiple levels of components. It provides a way to share values between components without having to explicitly pass a prop through every level of the tree. This is particularly useful for global data like user authentication status, theme settings, or language preferences, which need to be accessed by many components.
To understand how the Context API works, let’s break down its main components and steps involved in its implementation.
Creating a Context
The first step in using the Context API is to create a context. This is done using the React.createContext()
method. When you create a context, you can optionally pass in a default value, which will be used if a component does not have a matching provider above it in the tree.
const MyContext = React.createContext(defaultValue);
The defaultValue
is what consumers of the context will use if they do not have a Provider
wrapping them. This is useful for testing components in isolation without wrapping them in a provider.
Providing the Context
Once you have a context, the next step is to provide it to the components that need access to it. This is done using the Provider
component that comes with every context. The Provider
component accepts a value
prop, which is the data you want to make available to all components that consume this context.
<MyContext.Provider value={/* some value */}>
<MyComponent />
</MyContext.Provider>
Any component wrapped in MyContext.Provider
can now access the context value. Typically, the Provider
is placed high in the component tree, often at the root level, so that the context is available throughout the application.
Consuming the Context
To access the context value in a component, you use the useContext
hook or the Consumer
component. The useContext
hook is the most straightforward way to consume context and is used in functional components.
import React, { useContext } from 'react';
function MyComponent() {
const contextValue = useContext(MyContext);
return <div>{contextValue}</div>;
}
The useContext
hook takes the context object as its argument and returns the current context value.
For class components, or if you prefer a render prop pattern, you can use the Consumer
component.
<MyContext.Consumer>
{value => /* render something based on the context value */}
</MyContext.Consumer>
The Consumer
component requires a function as its child. This function receives the current context value and returns a React node.
Example: Theme Context
Let’s create a simple example to demonstrate how to use the Context API. We will create a theme context that allows us to switch between light and dark themes.
// ThemeContext.js
import React, { useState, createContext } from 'react';
export const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
In this example, we create a ThemeContext
and a ThemeProvider
component. The provider manages the theme state and provides a toggleTheme
function to switch between themes.
Now, we can use this context in our components:
// App.js
import React from 'react';
import { ThemeProvider } from './ThemeContext';
import ThemedComponent from './ThemedComponent';
function App() {
return (
<ThemeProvider>
<ThemedComponent />
</ThemeProvider>
);
}
export default App;
// ThemedComponent.js
import React, { useContext } from 'react';
import { ThemeContext } from './ThemeContext';
function ThemedComponent() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div style={{ background: theme === 'light' ? '#fff' : '#333', color: theme === 'light' ? '#000' : '#fff' }}>
<p>Current theme: {theme}</p>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
}
export default ThemedComponent;
In the ThemedComponent
, we consume the theme context using the useContext
hook. We use the context values to apply styles and toggle the theme when the button is clicked.
When to Use Context
The React Context API is a powerful tool, but it’s important to use it judiciously. Context is designed for global state management, where the state needs to be accessed by many components at different levels of the component tree. However, for state that is only relevant to a small part of the application, it’s better to keep it local to those components.
Overusing context can lead to performance issues, as every component that consumes the context will re-render whenever the context value changes. To mitigate this, you can split your context into smaller, more focused contexts, or use techniques like memoization to optimize performance.
Conclusion
The React Context API is a versatile solution for managing global state in React applications. By providing a way to share values across the component tree without prop drilling, it simplifies state management and improves code maintainability. Whether you’re managing themes, user authentication, or any other global data, the Context API can be a valuable addition to your React toolkit.
As you continue to build more complex applications, understanding and leveraging the Context API will become increasingly important. It’s a step towards building more scalable and maintainable React applications, allowing you to focus on what truly matters: delivering a great user experience.
Now answer the exercise about the content:
What problem does the React Context API solve in React development?
You are right! Congratulations, now go to the next page
You missed! Try again.
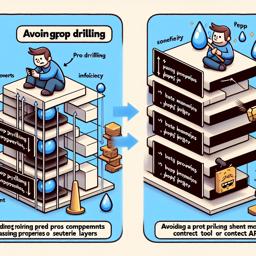
Next page of the Free Ebook: