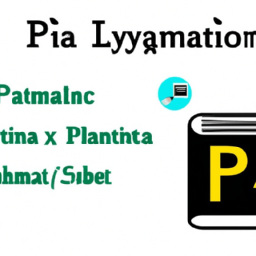
2.2. Python Language Fundamentals: Basic Python Syntax
Página 4
The Python programming language is known for its simplicity and clarity of syntax. It is a language that prioritizes code readability, making the learning process easier and more enjoyable for beginners. Furthermore, Python is a powerful and versatile language used in many areas of computing, including web development, data science, machine learning, among others.
To start programming in Python, you need to understand some fundamentals of the language, including its basic syntax. Syntax is the set of rules that define how Python programs are structured. Let's explore some fundamental aspects of Python syntax.
Indentation
In Python, indentation is used to delimit blocks of code. Unlike other programming languages that use braces ({}) for this purpose, Python uses whitespace. This means that code that is at the same indentation level is part of the same block. For example:
def hello_world(): print("Hello, world!")
In this example, the hello_world() function contains a block of code that is indented with four spaces. This block of code is executed when the function is called.
Comments
Comments in Python are lines that are not executed by the interpreter. They are used to explain what the code does, making it easier to understand. In Python, comments are created using the # symbol. For example:
# This is a comment print("Hello, world!") # This is also a comment
In this example, lines starting with # are comments and are not executed by the Python interpreter.
Variables and Data Types
Python is a dynamically typed programming language, which means that it is not necessary to declare the type of a variable when it is created. Python supports several data types, including integers, floating-point numbers, strings, and booleans. For example:
x = 10 # x is an integer y = 3.14 # y is a floating point number z = "Hello, world!" # z is a string a = True # a is a boolean
In this example, x, y, z and a are variables that store different types of data.
Operators
Python supports several operators, including arithmetic operators, comparison operators, and logical operators. Arithmetic operators include addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**). Comparison operators include equals (==), not equal (!=), less than (<), greater than (>), less than or equal (<=), and greater than or equal (>=). Logical operators include and (and), or (or), and not (not).
Flow Control
Python supports several control flow statements, including if, else, elif, for, and while. These instructions are used to control the order in which the code is executed. For example:
if x > 10: print("x is greater than 10") elif x < 10: print("x is less than 10") else: print("x is equal to 10")
In this example, the if statement checks whether x is greater than 10. If this is true, the code inside the if block is executed. If this is false, the elif statement checks whether x is less than 10. If this is true, the code inside the elif block is executed. If both conditions are false, the code inside the else block is executed.
These are just some of the fundamentals of the Python language. By mastering these concepts, you'll be well-prepared to explore the language's more advanced features, including functions, classes, modules, and more.
Now answer the exercise about the content:
Which of the following statements about the Python programming language is true?
You are right! Congratulations, now go to the next page
You missed! Try again.
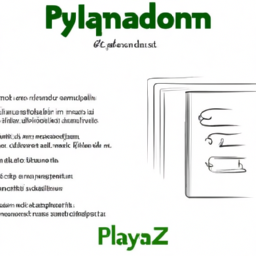
Next page of the Free Ebook: