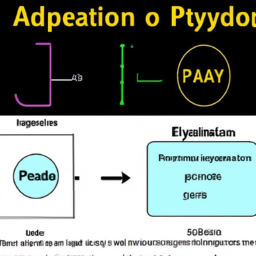
6.7. Object-Oriented Programming in Python: Static Methods in Python
Página 26
Object-oriented programming (OOP) is a programming paradigm that uses objects and their interactions to design applications and software programs. In Python, OOP plays a significant role as Python is an object-oriented programming language. In this context, we will discuss one of the most important concepts of OOP in Python - Static Methods.
Static methods in Python, as the name suggests, are methods that belong to a class and not a class instance. They cannot access or modify the state of the class, which means they cannot access or modify the instance or class variables. The main use of static methods is to group some utility functions related to the class.
To define a static method in Python, we use the @staticmethod decorator. A decorator is a function that modifies the functionality of another function or class. In Python, decorators are denoted with the @ symbol. To create a static method, we place the @staticmethod decorator before the method definition.
classMyClass: @staticmethod def my_static_method(): print("This is a static method.")
As you can see in the example above, we have defined a static method called my_static_method in the MyClass class. This method does not have the self parameter, which is a reference to the class instance. This is because static methods do not have access to the class instance or other instance or class variables.
To call a static method, we use the class name followed by the method name, as shown below:
MyClass.my_static_method()
This code will print "This is a static method." in the exit. Note that we do not need to create an instance of the class to call the static method.
Static methods are useful when we have some utility functions that are related to the class, but do not need to access or modify the state of the class. For example, we can have a Math class with a static method to calculate the factorial of a number. This method does not need to access or modify any instance or class variables, so it makes sense to make it a static method.
classMath: @staticmethod def factorial(n): if n == 0: return 1 else: return n * Math.factorial(n-1) print(Math.factorial(5)) # prints 120
In short, static methods in Python are methods that belong to a class and not a class instance. They cannot access or modify the state of the class, which means they cannot access or modify the instance or class variables. We use the @staticmethod decorator to define a static method. Static methods are useful when we have some utility functions that are related to the class, but do not need to access or modify the state of the class.
Understanding static methods is fundamental to object-oriented programming in Python, as they allow us to group class-related utility functions in a way that makes sense and is easy to understand. Therefore, when learning Python and object-oriented programming in Python, make sure you understand static methods well.
Now answer the exercise about the content:
What are static methods in Python and what is their role in object-oriented programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
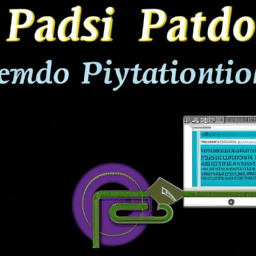
Next page of the Free Ebook: