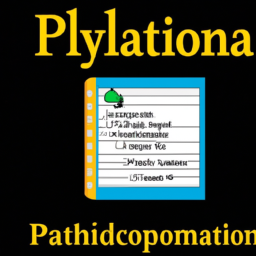
6.13. Object-Oriented Programming in Python: Exceptions in Object-Oriented Python
Página 32
In object-oriented programming in Python, exceptions play a crucial role in dealing with errors and unexpected situations that may occur during the execution of a program. Exceptions are events that can modify the control flow of a program. When an error occurs, or an exceptional condition is encountered in your program, Python creates an exception object. If you wrote code that handles the exception, the program can continue to execute and recover from the exception. Otherwise, the program terminates and Python prints a trace message.
To better understand exceptions in Python, let's first look at the concepts of try and except. The try statement works as follows: First, the try block (the code between the try and except statements) is executed. If no exception occurs, the except block is ignored and execution of the try statement is completed. If an exception occurs during the execution of the try block, the remainder of the block is ignored. If the corresponding exception type is found in the except clause, the except block is executed and then execution continues after the try statement.
For example:
try: x = 1 / 0 except ZeroDivisionError: print("You cannot divide by zero!")
In this case, we are trying to divide 1 by 0, which raises a ZeroDivisionError exception. Since we have an except block that handles this specific exception, the message "You cannot divide by zero!" is printed, rather than the program simply crashing.
Python has many built-in exception types that you can use in your code, such as ZeroDivisionError, TypeError, ValueError, etc. However, in some cases you may want to raise your own exceptions. To do this, you can use the raise keyword.
if x < 0: raise ValueError("The value of x cannot be negative")
Here, if x is less than zero, a ValueError exception is raised with the message "The value of x cannot be negative."
Also, in object-oriented Python, you can define your own exceptions by creating a new exception class. This class must inherit from an existing exception class, usually Exception or a subclass of it.
class MyException(Exception): pass raise MyException("This is a custom exception")
In this example, we create a new exception class called MyException that inherits from Exception. We then raise an instance of this exception with the message "This is a custom exception."
Exceptions are a fundamental part of Python programming and, when used correctly, can help make your code more robust and manageable. They allow you to handle errors and exceptional conditions elegantly, without breaking the execution of your program. At the same time, exceptions provide a way to flag problems that may occur, allowing you or other developers using your code to handle those problems appropriately.
In summary, object-oriented programming in Python with the use of exceptions allows you to create code that is robust, easy to debug, and capable of handling unexpected situations elegantly. If you are creating an API using Python, Lambda, and API Gateway, understanding exception handling in Python is essential to creating a robust and resilient API.
Now answer the exercise about the content:
What is the role of exceptions in object-oriented programming in Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
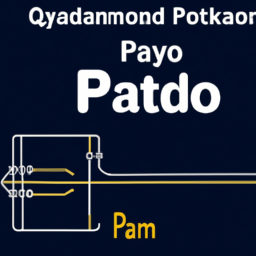
Next page of the Free Ebook: