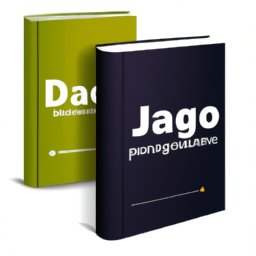
15.10. Models in Django: Views in Django
Página 84
Views in Django are the essential component of any Django application. They are responsible for processing HTTP requests and returning an HTTP response. In other words, Views are the interface between the application and the users. They are created in the views.py file within each Django application.
15.10.1. What are Views?
In Django, a View is a Python function that takes a web request and returns a web response. This response can be the HTML of a web page, a redirect, a 404 error, an XML document, an image or anything else that can be sent over HTTP. The View itself does not contain business logic, it just delegates to the model that is appropriate and displays the appropriate response.
15.10.2. How to create a View?
To create a View in Django, you need to define a function in views.py. This function must accept one argument: an HttpRequest object. And it should return an HttpResponse object. For example:
```python from django.http import HttpResponse def my_view(request): return HttpResponse("Hello World!") ```This is the simplest View possible in Django. To call the View, we need to map it to a URL - and for that we need a URLconf.
15.10.3. urlconf
To designate the URL that will lead to our View, we need to create a URL mapping. This is done in the urls.py file. The URL mapping is a table of contents for your Django application, containing a mapping between URLs and View functions.
For example:
```python from django.urls import path from .views import my_view urlpatterns = [ path('hello/', my_view), ] ```The /hello URL will now call the my_view function and display "Hello World!".
15.10.4. Views based on classes
Django also supports class-based Views. These are a more complex form of Views that are useful when your UI starts to reuse common code. Class-based Views allow you to structure your Views and reuse code by taking advantage of inheritance and mixing.
For example:
```python from django.views import View from django.http import HttpResponse class MyView(View): def get(self, request): return HttpResponse('Hello World!') ```To map a class-based View to a URL, you need to call the as_view() method in the URLconf:
```python from django.urls import path from .views import MyView urlpatterns = [ path('hello/', MyView.as_view()), ] ```Views in Django are a fundamental part of any Django application. They allow you to structure your application logically and reuse code where possible. Understanding how Views work is an essential step in becoming an effective Django developer.
15.10.5. Conclusion
Views in Django are a crucial component in the framework's MVC (Model-View-Controller) architecture. They work as a bridge between Models and Templates. Through Views, we can access data contained in Models, apply business logic and pass this data to Templates, where it will be rendered and presented to the user.
Views can be created either as simple functions or as classes, offering great flexibility for developers. Regardless of the chosen approach, it is important to remember that all Views must return an HttpResponse object, either directly or through a Template.
Finally, in order for a View to be accessible, it needs to be mapped to a specific URL through Django's URLconf system. This system allows you to define URL routes in a clear and organized way, facilitating the maintenance and scalability of the application.
Now answer the exercise about the content:
What is the function of Views in Django and how are they created?
You are right! Congratulations, now go to the next page
You missed! Try again.
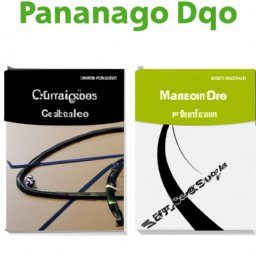
Next page of the Free Ebook: