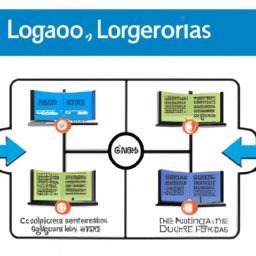
7.5. Logical Operators: Examples of using logical operators
Página 34
Logical operators are fundamental in programming logic, as they allow performing logical operations on Boolean values, which result in a true (true) or false (false) value. There are three main logical operators: AND (&&), OR (||), and NOT (!). Let's explore each of them with detailed examples.
AND Operator (&&)
The AND operator (&&) returns true if both operands are true. Consider the following example:
var a = true; var b = false; console.log(a && b); // returns false
In the example above, the variable 'a' is true and 'b' is false. Since the AND operator requires both operands to be true, the expression returns false.
OR operator (||)
The OR operator (||) returns true if at least one of the operands is true. See the following example:
var a = true; var b = false; console.log(a || b); // returns true
In the example above, the variable 'a' is true and 'b' is false. Since the OR operator requires at least one of the operands to be true, the expression returns true.
NOT operator (!)
The NOT operator (!) inverts the boolean value of the operand. If the operand is true, it will return false and vice versa. Here is an example:
var a = true; console.log(!a); // returns false
In the example above, the variable 'a' is true. The NOT operator reverses the value, so the expression returns false.
Examples of using logical operators
Logical operators are commonly used in control-of-flow conditions, such as if statements, while loops, and for loops. Here are some examples:
var a = 5; var b = 10; // Using the AND operator if (a > 0 && b > 0) { console.log("Both numbers are positive"); } // Using the OR operator if (a > 0 || b > 0) { console.log("At least one of the numbers is positive"); } // Using the NOT operator if (! (a > 0)) { console.log("A is not a positive number"); }
Logical operators are also useful for combining conditions. For example, you can use the AND operator to check whether two conditions are true at the same time. Or you can use the OR operator to check if at least one of the conditions is true.
In short, logical operators are powerful tools that allow you to manipulate Boolean values and control program flow. They are fundamental to programming logic and are used in almost all programs.
Now answer the exercise about the content:
What is the role of logical operators in programming and how do they work?
You are right! Congratulations, now go to the next page
You missed! Try again.
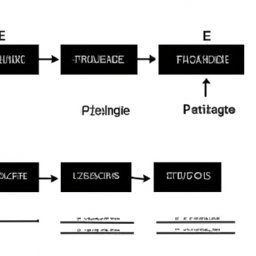
Next page of the Free Ebook: