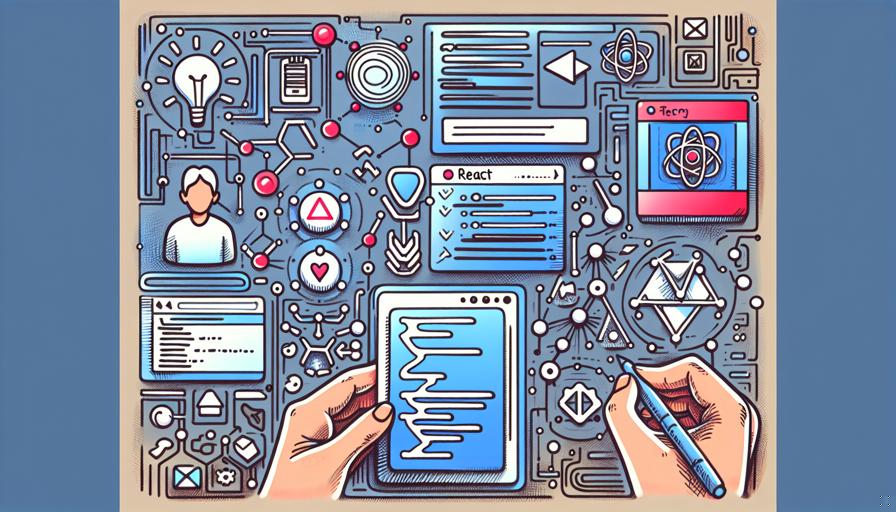
19. Handling Forms and User Input
Page 63 | Listen in audio
Handling forms and user input in React is a fundamental skill that every React developer needs to master. Forms are a crucial part of any web application as they allow users to interact with the app, submit information, and perform actions based on the data input. In React, managing forms and user input is slightly different from traditional HTML forms, due to its component-based architecture and the unidirectional data flow. This section will dive deep into the intricacies of form handling in React, covering controlled and uncontrolled components, form validation, and best practices.
In React, forms can be handled using two main approaches: controlled components and uncontrolled components. Understanding the difference between these two is essential for efficient form management.
Controlled Components
Controlled components are React components that render form elements and control their values via React state. In this approach, the form data is handled by the React component itself. The component maintains the state of the input fields and updates the state on every change. This approach provides a single source of truth for form data, making it easier to manage and validate input.
Here's a simple example of a controlled component in React:
{`
import React, { useState } from 'react';
function ControlledForm() {
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
alert('Submitted value: ' + inputValue);
};
return (
);
}
export default ControlledForm;
`}
In this example, the inputValue
state is used to store the value of the input field. The handleChange
function updates the state whenever the input changes, and the handleSubmit
function handles the form submission.
Uncontrolled Components
Uncontrolled components, on the other hand, rely on the DOM to store form data. Instead of using React state, you use a ref
to access the input values. This approach is more similar to traditional HTML form handling, where form data is handled by the DOM itself, and you only access it when needed.
Here's an example of an uncontrolled component:
{`
import React, { useRef } from 'react';
function UncontrolledForm() {
const inputRef = useRef(null);
const handleSubmit = (event) => {
event.preventDefault();
alert('Submitted value: ' + inputRef.current.value);
};
return (
);
}
export default UncontrolledForm;
`}
In this example, a ref
is created using the useRef
hook and assigned to the input element. The current value of the input is accessed via inputRef.current.value
during form submission.
Form Validation
Form validation is crucial to ensure that users provide the correct data. In React, you can perform validation either on the client-side or server-side, or both. Client-side validation is typically done using JavaScript to provide immediate feedback to the user, while server-side validation is performed after form submission to ensure data integrity.
For client-side validation, you can use functions to check the validity of input fields before allowing the form to be submitted. Here's an example of a simple validation in a controlled component:
{`
import React, { useState } from 'react';
function ValidatedForm() {
const [inputValue, setInputValue] = useState('');
const [error, setError] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
if (event.target.value.length < 3) {
setError('Name must be at least 3 characters long');
} else {
setError('');
}
};
const handleSubmit = (event) => {
event.preventDefault();
if (!error) {
alert('Submitted value: ' + inputValue);
}
};
return (
);
}
export default ValidatedForm;
`}
In this example, the handleChange
function checks if the input value is less than 3 characters long and sets an error message accordingly. The submit button is disabled if there is an error.
Best Practices for Form Handling in React
When handling forms in React, there are several best practices to follow:
- Use Controlled Components: Whenever possible, use controlled components for form handling. This approach provides better control over form data and makes it easier to implement validation and other features.
- Keep Form State Local: If the form state is only used within the form component, keep it local to avoid unnecessary re-renders of other components.
- Debounce Input Changes: For performance optimization, consider debouncing input changes, especially for inputs that trigger API calls or complex calculations.
- Use Libraries for Complex Forms: For complex forms with many fields and validation rules, consider using form libraries like Formik or React Hook Form. These libraries provide convenient APIs and features to simplify form handling.
- Provide User Feedback: Always provide feedback to users, such as validation messages or loading indicators, to improve the user experience.
In summary, handling forms and user input in React requires a good understanding of controlled and uncontrolled components, form validation techniques, and best practices. By mastering these concepts, you can build efficient and user-friendly forms that enhance the overall functionality of your React applications.
Now answer the exercise about the content:
What is the primary advantage of using controlled components for form handling in React?
You are right! Congratulations, now go to the next page
You missed! Try again.
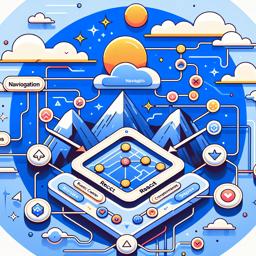
Next page of the Free Ebook: