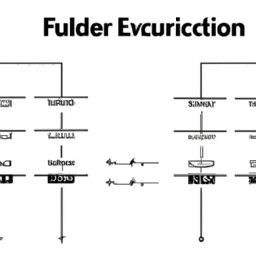
12. Flow control structures: repetition
Página 12
12. Flow Control Structures: Repetition
When learning programming logic, one of the key areas you need to understand is flow control structures. These structures are what give programs the ability to make decisions and repeat actions, making them useful and efficient. In this chapter, we will focus specifically on repeating flow control structures.
What are repeating flow control structures?
Repeating flow control structures, as the name suggests, are used to repeat a block of code multiple times. This is useful when you need to repeatedly perform an action, such as summing a list of numbers or fetching data from a database.
Types of repeating flow control structures
There are three main types of looping flow control structures: for, while, and do-while.
For
The for loop structure is used when you know exactly how many times you want a block of code to be executed. For example, if you wanted to add the numbers 1 to 10, you could use a for loop to do that. The for loop has three parts: the initialization, the condition, and the update. Initialization is where you set the initial counter value. The condition is the expression that determines whether or not the loop should continue. The update is where you change the counter value.
While
The while loop structure is used when you don't know how many times a block of code needs to be executed, but you know it should continue as long as a condition is true. For example, you can use a while loop to read data from a file until you reach the end. The while loop only has one part: the condition. If the condition is true, the code block will be executed. If it is false, the loop will terminate.
Do-While
The do-while loop structure is similar to while, but with one key difference: the block of code will be executed at least once, even if the condition is false from the start. This is useful when you need to perform an action at least once and then repeat it as long as a condition is true.
Examples of repeating flow control structures
Here are some examples of how you can use these repeating flow control structures in your code.
For
Suppose you want to print the numbers 1 to 10. You can do this using a for loop like this:
for (int i = 1; i <= 10; i++) { System.out.println(i); }
While
Suppose you want to read data from a file to the end. You can do this using a while loop like this:
while (!file.eof()) { String line = file.readLine(); System.out.println(line); }
Do-While
Suppose you want to prompt the user to enter a valid number. You can do this using a do-while loop like this:
of { System.out.println("Enter a number:"); number = scanner.nextInt(); } while (number <= 0);
Conclusion
The looping flow control structures are a fundamental part of programming logic. They allow you to run a block of code multiple times, which is useful in many different situations. By mastering these frameworks, you'll be one step closer to becoming an efficient and effective programmer.
Now answer the exercise about the content:
Which of the following statements correctly describes repeating flow control structures in programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
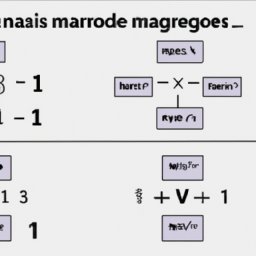
Next page of the Free Ebook: