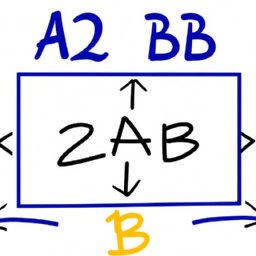
18. File manipulation
Page 18 | Listen in audio
File Manipulation in Logic Programming
In programming, file manipulation is an essential skill that allows programmers to read, write, update, and delete files. This chapter of our e-book course will focus on explaining in detail how to manipulate files, from basics to advanced.
What is File Manipulation?
File manipulation refers to the process of creating, reading, updating, and deleting files using a computer program. This is done using various functions and methods available in the standard libraries of the programming language you are using.
Why is File Manipulation Important?
File manipulation is a fundamental part of programming because it allows programs to interact with files on the computer's file system. This is useful for a variety of tasks, such as saving data for future use, reading data from existing files, and much more.
How to Manipulate Files
File handling varies from one programming language to another. However, there are some universal concepts you need to understand to be able to manipulate files effectively.
Opening Files
Before you can read or write to a file, you need to open it. This is usually done using a function like open()
or fopen()
, which returns a file object that you can use to read from or write to the file.
Reading Files
Once the file is open, you can read its contents using a function like read()
or fread()
. These functions usually return the contents of the file as a string, which you can then process as you wish.
Writing to Files
To write to a file, you can use a function like write()
or fwrite()
. These functions accept a string and write it to the file. If the file already contains data, these functions usually overwrite the existing data.
File Closing
Once you've finished reading or writing to a file, it's important to close it using a function like close()
or fclose()
. This frees up resources that the operating system has reserved for handling the file.
Practical Example
Let's consider a practical example of file manipulation in Python:
# Opening the file
file = open("example.txt", "w")
# Write to file
file.write("Hello World!")
# Closing the file
file.close()
This simple example opens a file called "example.txt" for writing, writes the string "Hello World!" in the file, and then close the file.
Conclusion
File manipulation is an essential programming skill that allows programs to interact with the computer's file system. Learning to handle files correctly is an important step in becoming an effective programmer.
In the next chapter of our e-book course, we'll explore more advanced concepts in file manipulation, including reading and writing binary files, manipulating large files, and much more. Stay tuned!
Now answer the exercise about the content:
What is file manipulation in programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
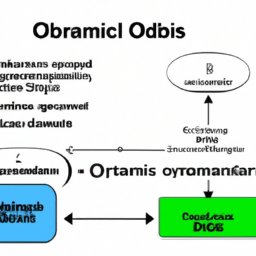
Next page of the Free Ebook: