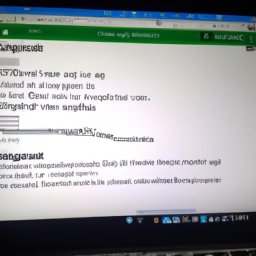
16.2. Creating a CRUD with NodeJS and MongoDB: MongoDB Installation
Página 86
Before we proceed with creating a CRUD (Create, Read, Update, Delete) with NodeJS and MongoDB, it is essential to understand what MongoDB is. MongoDB is a document-oriented NoSQL database. This means that instead of tables and rows as in relational databases, MongoDB is built around the concept of collections and documents.
To begin with, we need to install MongoDB on our system. Installation may vary depending on the operating system you are using. For this guide, we will assume that you are using a Unix-based operating system such as Ubuntu.
First, we need to update our system's package index with the following command:
sudo apt-get update
Next, we can install MongoDB with:
sudo apt-get install -y mongodb
Once the installation is complete, you can start MongoDB with:
sudo service mongodb start
To verify that the installation was successful, you can run:
sudo service mongodb status
Now that we have MongoDB installed, we can start creating our CRUD with NodeJS. First, we need to install NodeJS on our system. You can do this with the following command:
sudo apt-get install -y nodejs
After installing NodeJS, we need to install NPM (Node Package Manager), which is a package manager for NodeJS. You can do this with the following command:
sudo apt-get install -y npm
Now that we have NodeJS and NPM installed, we can start creating our project. First, let's create a new directory for our project and navigate to it with:
mkdir my-api && cd my-api
Next, let's initialize a new NodeJS project with:
npm init -y
This will create a new package.json file in our directory, which will contain our project information and its dependencies.
To interact with MongoDB, we need to install a NodeJS driver for MongoDB. The most popular is Mongoose, which we can install with:
npm install mongoose
Now we are ready to start writing our CRUD. First, let's create a new file called app.js in our directory with:
touch app.js
Next, let's open this file in our preferred text editor and start writing our code. First, we need to import Mongoose with:
const mongoose = require('mongoose');
Next, we need to connect Mongoose to our MongoDB database with:
mongoose.connect('mongodb://localhost/my_database', {useNewUrlParser: true, useUnifiedTopology: true});
Now we are ready to define our database schema and create our CRUD functions. A schema in Mongoose is a structure that defines the shape of documents within a collection. We can define a schema with:
const UserSchema = new mongoose.Schema({ name: String, email: String, password: String });
We can then create a model from this schema with:
const User = mongoose.model('User', UserSchema);
Now we can create our CRUD functions. For example, to create a new user, we can do:
const user = new User({ name: 'John', email: '[email protected]', password: 'secret' }); user.save();
To read a user, we can do:
User.findById('user_id');
To update a user, we can do:
User.findByIdAndUpdate('user_id', { name: 'Jane' });
And to delete a user, we can do:
User.findByIdAndDelete('user_id');
With this, you should have a basic understanding of how to create a CRUD with NodeJS and MongoDB. Keep in mind that this is just a basic example and you can expand on this to suit your specific needs.
Now answer the exercise about the content:
What is MongoDB and how is it structured?
You are right! Congratulations, now go to the next page
You missed! Try again.
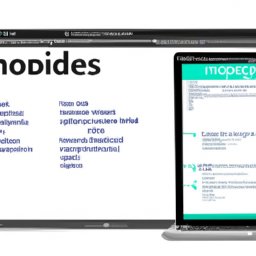
Next page of the Free Ebook: