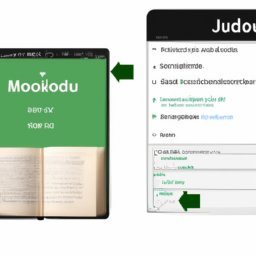
16.8. Creating a CRUD with NodeJS and MongoDB: Implementing functions to create, read, update and delete data
Página 92
16.8. Creating a CRUD with NodeJS and MongoDB: Implementation of functions to create, read, update and delete data
For software developers, the ability to create, read, update, and delete data (CRUD) is an essential skill. In the context of our e-book course on how to create APIs in NodeJS, we will now focus on how to implement these operations using NodeJS and MongoDB.
Configuring the Environment
Before we get started, it's important to make sure you have the right development environment. You will need NodeJS and MongoDB installed on your system. In addition, we will use Express, a framework for NodeJS, and Mongoose, an ORM (Object Relational Mapping) for MongoDB.
Creating the Data Model
First, we need to define our data model. Let's assume we are creating an API for a blog application. So our data model could be a 'Post', with properties like 'title', 'content' and 'author'. Using Mongoose, we can define this model like this:
const mongoose = require('mongoose'); const Schema = mongoose.Schema; const PostSchema = new Schema({ title: String, content: String, author: String }); module.exports = mongoose.model('Post', PostSchema);
Implementing CRUD Operations
Create
Let's start with the 'Create' operation. We will use the POST route for this. Here is an example of how this can be done:
const express = require('express'); const router = express.Router(); const Post = require('../models/Post'); router.post('/', async (req, res) => { const post = new Post({ title: req.body.title, content: req.body.content, author: req.body.author }); try { const savedPost = await post.save(); res.json(savedPost); } catch (err) { res.json({ message: err }); } });
Read (Read)
Next, let's implement the 'Read' operation. We will use the GET route for this. Here is an example of how to read all posts and a specific post:
// Get All Posts router.get('/', async (req, res) => { try { const posts = await Post.find(); res.json(posts); } catch (err) { res.json({ message: err }); } }); // Get Specific Post router.get('/:postId', async (req, res) => { try { const post = await Post.findById(req.params.postId); res.json(post); } catch (err) { res.json({ message: err }); } });
Update
Now, let's implement the 'Update' operation. We will use the PATCH route for this. Here is an example of how to update a specific post:
router.patch('/:postId', async (req, res) => { try { const updatedPost = await Post.updateOne( { _id: req.params.postId }, { $set: { title: req.body.title, content: req.body.content, author: req.body.author } } ); res.json(updatedPost); } catch (err) { res.json({ message: err }); } });
Delete (Delete)
Finally, let's implement the 'Delete' operation. We will use the DELETE route for this. Here is an example of how to delete a specific post:
router.delete('/:postId', async (req, res) => { try { const removedPost = await Post.remove({ _id: req.params.postId }); res.json(removedPost); } catch (err) { res.json({ message: err }); } });
Conclusion
With that, we complete the implementation of CRUD operations using NodeJS and MongoDB. The beauty of NodeJS is its simplicity and efficiency, and with the help of packages like Express and Mongoose, we can create powerful and scalable APIs. Remember, practice makes perfect, so keep practicing and experimenting.
Now answer the exercise about the content:
In the context of the e-book course presented in the text, what is the purpose of the functions to create, read, update and delete data in NodeJS and MongoDB?
You are right! Congratulations, now go to the next page
You missed! Try again.
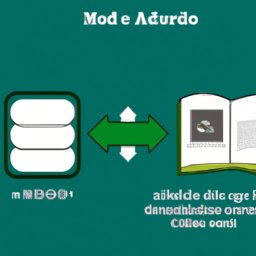
Next page of the Free Ebook: