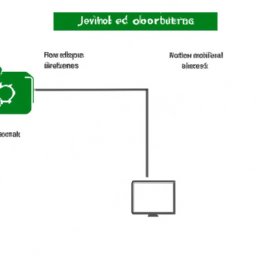
5.6. Creating a basic server with NodeJS: Working with HTTP requests
Página 37
5.6. Creating a basic server with NodeJS: Working with HTTP requests
NodeJS is a JavaScript application development platform that allows you to build scalable, high-performance web applications. One of the most outstanding features of NodeJS is the ability to create HTTP servers quickly and easily. In this chapter, we are going to learn how to create a basic server with NodeJS and work with HTTP requests.
NodeJS Installation
Before you start, you need to install NodeJS on your operating system. You can download the latest version of NodeJS from the official website (https://nodejs.org). After downloading, follow the installation instructions provided by the installer. To verify that the installation was successful, open the terminal and enter the following command: node -v. If the installation is successful, the terminal will display the version of NodeJS installed on your system.
Creating a basic server with NodeJS
To create a basic server with NodeJS, you need to create a JavaScript file and write a few lines of code. Let's create a file called server.js and add the following code:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
This code creates an HTTP server that listens on port 3000. When a request is received, the server responds with HTTP status 200 (OK) and the text "Hello World".
Working with HTTP requests
When creating a server with NodeJS, you can handle HTTP requests in several ways. In the example above, we just responded with plain text. However, you can send files, JSON, HTML and more.
To handle HTTP requests, you can use the request object (req) that is passed to the callback function of the createServer method. This object contains information about the HTTP request, such as the HTTP method, URL, headers and body of the request.
For example, you can check the HTTP method of the request as follows:
const http = require('http');
const server = http.createServer((req, res) => {
console.log(req.method);
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
This code will display the HTTP method of the request in the console. If you access the server with a browser, the HTTP method will be GET.
You can also check the URL of the request. For example, you can respond differently based on the request URL as follows:
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/about') {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('About\n');
} else {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
}
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
This code will respond with "About" if the request URL is "/about". Otherwise, it will respond with "Hello World".
In summary, creating a basic server with NodeJS is simple and fast. Furthermore, NodeJS provides a powerful API to handle HTTP requests, which allows you to create complex and scalable web applications.
Now answer the exercise about the content:
Which of the following statements is true about building a basic server with NodeJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
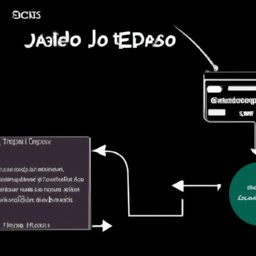
Next page of the Free Ebook: