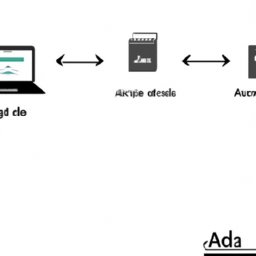
5.12. Creating a Basic Server with NodeJS: Testing and Documenting the API
Página 43
5.12. Creating a Basic Server with NodeJS: Testing and Documenting the API
Developing an API in NodeJS involves several steps, from setting up the environment to implementing routes and tests. In this chapter, we'll focus on creating a basic server in NodeJS and how to test and document the API.
Configuring the Server
The first step is to configure the server. For that, we need to install NodeJS and NPM (Node Package Manager) on our system. After installation, we create a new directory for our project and initialize a new NodeJS project with the
npm init
command. This will create a package.json
file, which will be used to manage our project's dependencies.
Now, let's install Express, a framework for NodeJS that simplifies web server development. To install Express, we use the command
npm install express
.
With Express installed, we can create our basic server. We create a new file called server.js
and in it, we import Express and start a new application. Next, we define a basic route that responds with a "Hello, World!" when accessed.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Testing the API
With the server created, the next step is to test the API. For this, we will use Postman, a tool that allows you to send HTTP requests to a server and view the responses.
To test the route we created, we open Postman, select the GET method, enter the server URL (http://localhost:3000) and click "Send". If everything is working correctly, we should see a "Hello, World!" in the answer.
Documenting the API
Documentation is a crucial part of any API. It allows other developers to understand how to use the API and what features are available. Documentation should include information about routes, supported HTTP methods, expected parameters, and possible status codes and responses.
There are several tools available for documenting APIs, such as Swagger and API Blueprint. These tools allow you to create interactive documentation that can be easily updated as the API evolves. To use Swagger, for example, we need to install the swagger-ui-express
module and create a JSON file with the API specification.
With all these steps completed, we have a basic NodeJS server with a tested and documented API. However, this is just the beginning. As the API grows, we'll need to add authentication, handle errors, implement automated tests, and more. But with a solid foundation, we are well prepared to meet these challenges.
Now answer the exercise about the content:
What is the process for developing a basic API in NodeJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
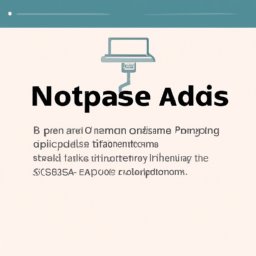
Next page of the Free Ebook: