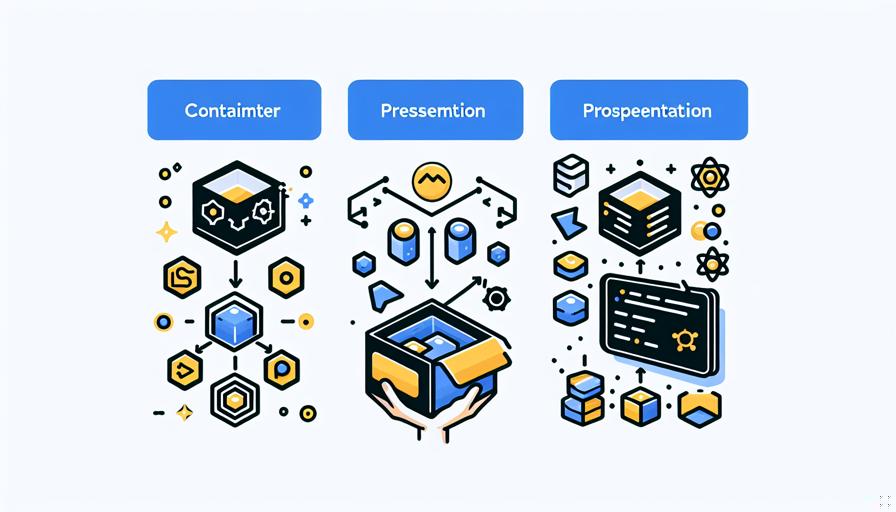
6.2. Components: Container vs Presentation Components
Page 8 | Listen in audio
React JS, a popular library for building user interfaces, introduces a component-based architecture that allows developers to build reusable UI elements. One of the fundamental concepts in React development is the distinction between Container and Presentation Components. Understanding this distinction is crucial for structuring your applications effectively and maintaining a clean separation of concerns.
In the early days of React, Dan Abramov, one of the core contributors to the library, introduced the idea of splitting components into two categories: Container and Presentation Components. This pattern helps in organizing components based on their responsibilities and roles within an application.
Presentation Components
Presentation Components, sometimes referred to as "Dumb" components, are primarily concerned with how things look. They are responsible for rendering UI elements and are generally stateless. These components receive data and callbacks exclusively through props, making them easy to test and reuse across different parts of an application. Their simplicity and lack of side effects make them predictable and easy to reason about.
Some key characteristics of Presentation Components include:
- Stateless: They do not manage their own state. Any data they require is passed to them through props.
- UI-focused: Their primary responsibility is to render HTML and CSS. They determine the look and feel of the application.
- Reusable: Because they are not tied to any specific application logic, they can be reused across different parts of an application or even in different projects.
- Functional Components: Often implemented as functional components, which makes them more concise and easier to understand.
Here's an example of a simple Presentation Component:
function Button({ label, onClick }) {
return (
<button onClick={onClick}>
{label}
</button>
);
}
In this example, the Button
component is purely focused on rendering a button element with a label. It does not know where the label comes from or what happens when the button is clicked. It simply receives these details via props.
Container Components
Container Components, sometimes called "Smart" components, are concerned with how things work. They manage state and handle the logic of the application. These components are responsible for fetching data, managing state changes, and passing data down to Presentation Components. They act as the brains of the application, orchestrating the flow of data and interactions.
Some key characteristics of Container Components include:
- Stateful: They often maintain their own state and manage data fetching and updates.
- Logic-focused: Responsible for handling business logic, data manipulation, and communication with APIs or other services.
- Less Reusable: Due to their specific logic and state management, they are less reusable compared to Presentation Components.
- Class Components or Hooks: Traditionally implemented as class components, but with the advent of hooks, they can also be functional components using
useState
,useEffect
, and other hooks.
Here's an example of a simple Container Component:
import React, { useState, useEffect } from 'react';
import Button from './Button';
function ButtonContainer() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log(`Count has been updated to: ${count}`);
}, [count]);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<Button label="Increment" onClick={increment} />
</div>
);
}
In this example, the ButtonContainer
component manages the state of the count and passes the necessary props to the Button
Presentation Component. It handles the logic of incrementing the count and logging updates, while the Button
component is solely responsible for rendering the button.
Benefits of Separating Components
Separating components into Container and Presentation Components offers several benefits:
- Separation of Concerns: By dividing components based on their responsibilities, you achieve a clear separation of concerns, making the codebase easier to understand and maintain.
- Improved Reusability: Presentation Components can be reused across different parts of the application or even in different projects, reducing duplication and improving consistency.
- Enhanced Testability: Presentation Components, being stateless and focused on rendering, are easier to test. You can test them in isolation without worrying about the underlying logic.
- Scalability: As the application grows, maintaining a clear distinction between UI and logic components helps in scaling the codebase, making it easier to manage and extend.
Implementing the Pattern
While the Container and Presentation Components pattern is a useful guideline, it is not a strict rule. In practice, you might find components that blur the lines between these categories. The key is to focus on the responsibilities of each component and strive for a clean separation of concerns.
With the introduction of hooks in React, the lines between Container and Presentation Components have become more flexible. Hooks allow you to share logic between components without changing their hierarchy, making it easier to maintain a separation of concerns while using functional components.
For example, you can create custom hooks that encapsulate specific logic, allowing you to use them in both Container and Presentation Components. This approach provides flexibility in how you structure your components while maintaining the benefits of the pattern.
Conclusion
The distinction between Container and Presentation Components is a powerful concept in React development. By organizing components based on their responsibilities, you can create a more maintainable, scalable, and testable codebase. While the pattern is not a strict rule, it serves as a valuable guideline for structuring your React applications effectively.
As you gain experience with React, you'll develop an intuition for when to apply this pattern and when to adapt it to suit your specific needs. By embracing the principles of separation of concerns and focusing on the roles of your components, you can build robust and maintainable applications with React JS.
Now answer the exercise about the content:
What is the primary responsibility of Presentation Components in React JS?
You are right! Congratulations, now go to the next page
You missed! Try again.
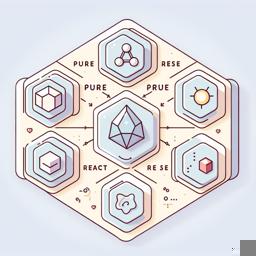
Next page of the Free Ebook: