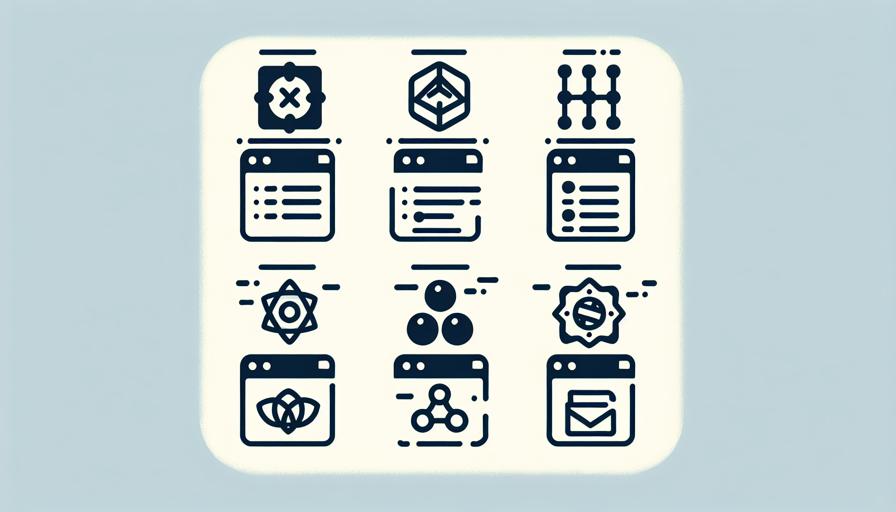
6.12. Components: Component Style Management
Page 18 | Listen in audio
In the world of React, components are the building blocks that allow developers to create complex and dynamic user interfaces. As your React applications grow, managing the styles of these components becomes crucial. Effective component style management not only ensures that your application looks consistent but also enhances maintainability and scalability. In this section, we'll explore various strategies and tools for managing component styles in React.
Inline Styles
One of the simplest ways to apply styles to a React component is through inline styles. Inline styles are defined directly within a component using a JavaScript object. This approach allows you to dynamically calculate styles based on component state or props.
function Button({ primary }) {
const style = {
backgroundColor: primary ? 'blue' : 'gray',
color: 'white',
padding: '10px 20px',
border: 'none',
borderRadius: '5px',
};
return ;
}
While inline styles are straightforward and easy to use, they have limitations. They do not support pseudo-classes (like :hover
) or media queries directly. For complex styling needs, other approaches might be more suitable.
CSS Stylesheets
Traditional CSS stylesheets are another common method for styling React components. You can create a separate CSS file, define your styles, and then import it into your component file. This approach keeps your styles separate from your JavaScript code, promoting separation of concerns.
// Button.css
.button {
background-color: gray;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
}
.button.primary {
background-color: blue;
}
// Button.js
import './Button.css';
function Button({ primary }) {
return ;
}
Using CSS stylesheets provides more flexibility, allowing you to leverage CSS features like media queries, pseudo-classes, and keyframe animations. However, it can lead to global namespace conflicts if not managed properly.
CSS Modules
CSS Modules offer a solution to the global namespace issue by automatically scoping styles to the component. When you use CSS Modules, each class name is locally scoped by default, preventing conflicts with other components.
// Button.module.css
.button {
background-color: gray;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
}
.primary {
background-color: blue;
}
// Button.js
import styles from './Button.module.css';
function Button({ primary }) {
return ;
}
CSS Modules are a powerful tool for managing component styles, especially in large applications. They provide the benefits of traditional CSS while avoiding the pitfalls of global scope.
Styled Components
Styled Components is a popular library that brings the power of CSS-in-JS to React. It allows you to write CSS directly within your JavaScript files, creating styled components. This approach promotes component-based styling and encapsulation.
import styled from 'styled-components';
const Button = styled.button`
background-color: ${props => (props.primary ? 'blue' : 'gray')};
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
`;
function App() {
return ;
}
Styled Components offer several advantages, including:
- Automatic scoping of styles to components, preventing global conflicts.
- Dynamic styling based on props and state, making it easy to create responsive designs.
- Full support for CSS features, including media queries and pseudo-classes.
However, Styled Components can introduce a runtime overhead, as styles are generated and injected during component rendering. It's important to consider performance implications, especially in performance-critical applications.
Emotion
Emotion is another CSS-in-JS library that offers a flexible and performant way to style React components. It provides a similar API to Styled Components but with additional features like css
prop and Global
styles.
/** @jsxImportSource @emotion/react */
import { css } from '@emotion/react';
const buttonStyle = css`
background-color: gray;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
`;
function Button({ primary }) {
return (
);
}
Emotion allows you to write styles with the full power of JavaScript, enabling advanced dynamic styling scenarios. It also supports server-side rendering and has a smaller bundle size compared to some other CSS-in-JS libraries.
Choosing the Right Approach
When it comes to choosing a style management approach for your React components, there is no one-size-fits-all solution. The choice depends on various factors, including project requirements, team preferences, and performance considerations. Here are some guidelines to help you make an informed decision:
- Project Size: For small projects, inline styles or traditional CSS stylesheets might suffice. As the project grows, consider CSS Modules or CSS-in-JS solutions for better scalability.
- Component Encapsulation: If component encapsulation and avoiding global style conflicts are priorities, CSS Modules or CSS-in-JS libraries like Styled Components and Emotion are excellent choices.
- Dynamic Styling: If your components require a lot of dynamic styling based on props or state, CSS-in-JS solutions offer the flexibility needed to achieve this.
- Performance: Consider the performance implications of CSS-in-JS libraries, especially in large applications. Evaluate the trade-offs between style encapsulation and runtime overhead.
- Team Familiarity: Choose an approach that aligns with your team's expertise and preferences. If your team is already familiar with CSS-in-JS libraries, leveraging them can lead to faster development and better maintainability.
Ultimately, the goal is to choose a style management strategy that aligns with your project's needs and enhances the development experience. As you gain experience with different approaches, you'll be better equipped to make informed decisions about style management in your React applications.
In conclusion, component style management is a critical aspect of building React applications. By understanding and leveraging the various styling options available, you can create well-structured, maintainable, and visually appealing user interfaces. Whether you choose inline styles, CSS stylesheets, CSS Modules, or CSS-in-JS libraries, each approach has its strengths and trade-offs. The key is to find the right balance that suits your project's requirements and development workflow.
Now answer the exercise about the content:
Which styling approach in React is described as allowing you to write CSS directly within your JavaScript files, promoting component-based styling and encapsulation?
You are right! Congratulations, now go to the next page
You missed! Try again.
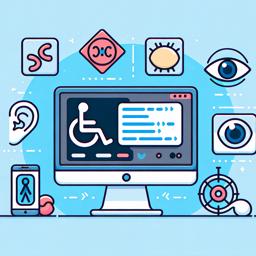
Next page of the Free Ebook: