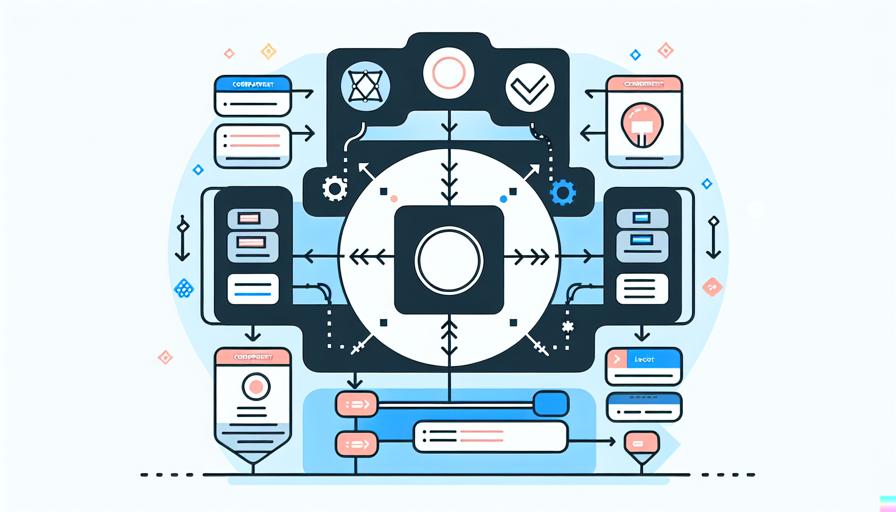
6.8. Components: Component Communication Patterns
Page 14 | Listen in audio
In React, components are the fundamental building blocks of an application. They encapsulate a piece of the user interface and its associated logic, creating a modular and reusable structure. However, as applications grow in complexity, the need for these components to communicate with each other becomes crucial. Understanding component communication patterns is essential for building scalable and maintainable React applications.
React components communicate through a unidirectional data flow, meaning data flows in one direction, from parent to child. This approach simplifies the data flow and makes it easier to understand how data changes affect the UI. However, there are several patterns and techniques that developers can use to facilitate communication between components.
Props: The Primary Communication Channel
Props are the primary mechanism for passing data and event handlers from parent to child components. When a parent component renders a child component, it can provide data to the child via props. This allows the child to display dynamic content based on the data it receives.
function ParentComponent() {
const data = "Hello from Parent!";
return <ChildComponent message={data} />;
}
function ChildComponent({ message }) {
return <h1>{message}</h1>;
}
In this example, the ParentComponent
passes a message prop to the ChildComponent
, which then renders it. This pattern is simple and effective for many scenarios, especially when the data flow is straightforward.
Callback Functions: Communicating Upwards
While props allow data to flow downwards, callback functions enable child components to communicate with their parents. By passing a function as a prop, a parent component can allow a child component to notify it of events or changes.
function ParentComponent() {
const handleChildClick = () => {
alert("Child component clicked!");
};
return <ChildComponent onClick={handleChildClick} />;
}
function ChildComponent({ onClick }) {
return <button onClick={onClick}>Click Me</button>;
}
In this example, the ParentComponent
passes a function handleChildClick
to the ChildComponent
. When the button in the child component is clicked, it calls this function, allowing the parent to respond to the event.
Context API: Avoiding Prop Drilling
As applications grow, passing props through multiple levels of nested components can become cumbersome, a problem known as "prop drilling." The Context API offers a solution by allowing components to share values without explicitly passing props through every level of the component tree.
const ThemeContext = React.createContext("light");
function App() {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
return (
<div>
<ThemedButton />
</div>
);
}
function ThemedButton() {
const theme = React.useContext(ThemeContext);
return <button className={theme}>Themed Button</button>;
}
In this example, the ThemeContext
provides a way to pass the theme value throughout the component tree without having to pass it explicitly through props. The ThemedButton
component can access the theme value directly using the useContext
hook.
State Management Libraries: Handling Complex State
For applications with complex state management needs, libraries like Redux or MobX can be used to manage state across the application. These libraries provide a centralized store for application state and allow components to subscribe to changes in the state.
Redux Example
// actions.js
export const increment = () => ({ type: "INCREMENT" });
// reducer.js
const initialState = { count: 0 };
function counterReducer(state = initialState, action) {
switch (action.type) {
case "INCREMENT":
return { count: state.count + 1 };
default:
return state;
}
}
// store.js
import { createStore } from "redux";
import counterReducer from "./reducer";
const store = createStore(counterReducer);
// CounterComponent.js
import { useSelector, useDispatch } from "react-redux";
import { increment } from "./actions";
function CounterComponent() {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch(increment())}>Increment</button>
</div>
);
}
In this Redux example, the global state is managed in a store, and components can dispatch actions to modify the state. The CounterComponent
uses hooks from the react-redux
library to access and update the state.
Custom Hooks: Encapsulating Logic
Custom hooks are a powerful feature in React that allow developers to encapsulate reusable logic. They can be used to share stateful logic across components without duplicating code.
function useWindowWidth() {
const [width, setWidth] = React.useState(window.innerWidth);
React.useEffect(() => {
const handleResize = () => setWidth(window.innerWidth);
window.addEventListener("resize", handleResize);
return () => window.removeEventListener("resize", handleResize);
}, []);
return width;
}
function DisplayWidthComponent() {
const width = useWindowWidth();
return <p>Window width: {width}px</p>;
}
In this example, the useWindowWidth
hook encapsulates the logic for tracking the window width, allowing any component to easily access this information without duplicating the logic.
Conclusion
React provides a variety of tools and patterns for component communication. By understanding and leveraging these patterns, developers can build applications that are both scalable and maintainable. Whether using props for simple data flow, the Context API to avoid prop drilling, or state management libraries for complex state, each pattern has its place in a React developer's toolkit.
As you continue to develop with React, you'll find that choosing the right communication pattern often depends on the specific requirements of your application and the complexity of your component hierarchy. Practice and experience will guide you in making these decisions, leading to more efficient and effective React applications.
Now answer the exercise about the content:
What is the primary mechanism for passing data and event handlers from parent to child components in React?
You are right! Congratulations, now go to the next page
You missed! Try again.
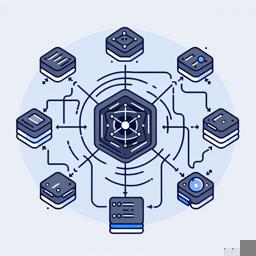
Next page of the Free Ebook: