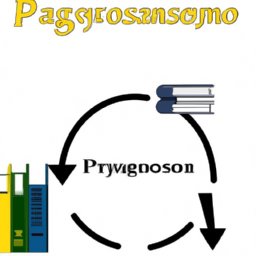
7.9. Classes and Objects in Python: Operator Overloading
Página 45
7.9. Python Classes and Objects: Operator Overloading
Python, being an object-oriented programming language, uses classes and objects to structure its code. A class is a template for creating objects, while an object is an instance of a class. In this chapter, we'll discuss operator overloading in Python, an advanced concept that allows operators to have different meanings depending on context.
Operator Overload
Operator overloading is a feature in object-oriented programming languages that allows operators like +, -, *, /, etc., to be used in different ways depending on the data types of the operands. In Python, this is done through special methods defined in classes. These methods begin and end with two underscores (__).
Operator Overloading Example
Consider the following class called 'Complex' that represents a complex number:
class Complex: def __init__(self, real, image): self.real = real self.imag = image
We can create two objects of this class and try to add them using the '+' operator. However, Python does not know how to add two 'Complex' objects and will return an error.
To solve this, we can define a special method called '__add__' in the 'Complex' class:
class Complex: def __init__(self, real, image): self.real = real self.imag = image def __add__(self, other): return Complex(self.real + other.real, self.imag + other.imag)
Now, when we try to add two 'Complex' objects, Python will call the '__add__' method we defined, allowing the operation to be performed correctly.
More on Special Methods
There are many other special methods that we can define in our classes to overload other operators. Some examples include '__sub__' for subtraction, '__mul__' for multiplication, '__truediv__' for division, '__mod__' for modulus, and so on.
In addition, we can also overload comparison operators like '==', '!=', '<', '>', '<=', and '>='. For that, we use special methods like '__eq__', '__ne__', '__lt__', '__gt__', '__le__', and '__ge__', respectively.
Final Considerations
Operator overloading is a powerful feature that allows our classes to behave more naturally and intuitively. However, it should be used with care as it can make the code harder to understand if used improperly.
Also, not all operators can be overloaded in Python. Some operators, like 'is', 'not', and 'and', have fixed behaviors that cannot be changed.
In summary, operator overloading is a useful tool to have in your Python programming arsenal, but like all tools, it should be used appropriately and responsibly.
Now answer the exercise about the content:
What is operator overloading in Python and how is it implemented?
You are right! Congratulations, now go to the next page
You missed! Try again.
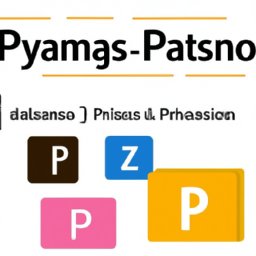
Next page of the Free Ebook: