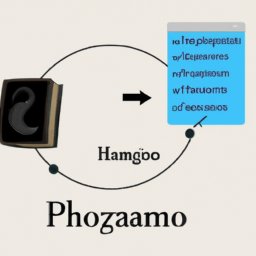
7.4. Classes and Objects in Python: Inheritance and Polymorphism
Página 40
Inheritance and polymorphism are two fundamental concepts in object-oriented programming (OOP) and are widely used in many programming languages, including Python. Let's explore these concepts in detail.
7.4.1 Inheritance
Inheritance is a feature of object-oriented programming that allows a class to inherit attributes and methods from another class. The class from which attributes and methods are inherited is called the base class or parent class, and the class that inherits these attributes and methods is called the child class or derived class.
In Python, inheritance is accomplished by defining a new class, followed by the parent class names in parentheses.
class ParentClass: pass class ChildClass(ParentClass): pass
Here, ChildClass
is a subclass of ParentClass
and inherits all of its attributes and methods.
Python also supports multiple inheritance, where a class can inherit from multiple parent classes. This is accomplished by listing all parent classes in parentheses, separated by commas.
class ParentClass1: pass class FatherClass2: pass class ChildClass(ParentClass1, ParentClass2): pass
Here, ChildClass
is a subclass of ParentClass1
and ParentClass2
and inherits all of their attributes and methods.
7.4.2 Polymorphism
Polymorphism is another fundamental feature of object-oriented programming. It allows an object to take many forms. More specifically, polymorphism allows a child class to share the same method name as its parent class and provide a different implementation of that method.
For example, consider two classes, ParentClass
and ChildClass
. Both classes have a method called example_method
. However, the implementation of this method in each class is different.
class ParentClass: def example_method(self): print("ParentClass Method") class ChildClass(ParentClass): def example_method(self): print("ChildClass Method")
Here, ChildClass
is a subclass of ParentClass
and both classes have a method called example_method
. However, the implementation of this method in each class is different. This is an example of polymorphism.
Python allows polymorphism in two ways: method overloading and method substitution. Method overloading allows a class to have multiple methods with the same name but different method signatures. Method override allows a child class to provide a different implementation of a method that is already provided by its parent class.
In summary, inheritance and polymorphism are fundamental features of object-oriented programming that allow code reuse and flexibility in implementing different object behaviors. They are widely used in many programming languages, including Python, and are key to building efficient, reusable systems.
Now answer the exercise about the content:
What are the two fundamental concepts in object-oriented programming (OOP) and how are they used in Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
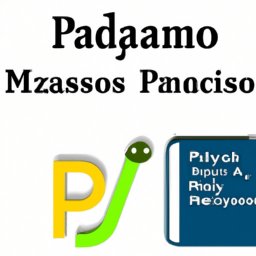
Next page of the Free Ebook: