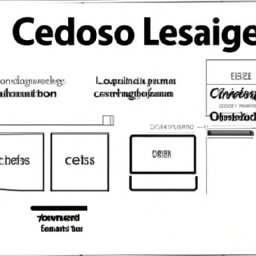
17.2. Classes and Objects: Attributes and methods of a class
Page 46 | Listen in audio
17.2. Classes and Objects: Attributes and Methods of a Class
In object-oriented programming, a class is a blueprint or blueprint that defines what an object can do. An object, on the other hand, is an instance of a class. In this chapter, we will explore the attributes and methods of a class, which are essential components in defining a class.
Attributes of a Class
Attributes are variables that belong to a class. They are used to represent the state of an object. For example, if we have a class called 'Car', some of the attributes we might have include 'color', 'model', 'year' and 'make'. These attributes help define the specific characteristics of an object.
The attributes of a class are defined in the constructor method of the class. The constructor method is a special method that is called automatically when an object is created. It is used to initialize an object's attributes.
For example, here is a 'Car' class with a constructor method that initializes the 'color', 'model', 'year' and 'make' attributes:
class Car { constructor(color, model, year, brand) { this.color = color; this.model = model; this.year = year; this.mark = mark; } }
Here, 'this' is a reference to the current object. It is used to access the object's attributes.
Methods of a Class
Methods are functions that belong to a class. They are used to define the behavior of an object. For example, in the 'Car' class, we can have methods like 'on', 'off', 'accelerate' and 'brake'. These methods define what a car can do.
The methods of a class are defined inside the body of the class, outside the constructor method. For example, here is the 'Car' class with the 'on', 'off', 'accelerate' and 'brake' methods:
class Car { constructor(color, model, year, brand) { this.color = color; this.model = model; this.year = year; this.mark = mark; } to connect() { console.log('The car is on'); } to switch off() { console.log('The car is off'); } speed up() { console.log('The car is accelerating'); } brake() { console.log('The car is braking'); } }
To call a method of an object, we use dot notation. For example, to call the 'power on' method on a 'car' object, we do 'car.power on()'.
Summary
In short, a class in object-oriented programming is a blueprint that defines what an object can do. A class is made up of attributes and methods. Attributes are variables that represent the state of an object, while methods are functions that define an object's behavior. Attributes of a class are initialized in the constructor method of the class, while methods are defined inside the body of the class, outside the constructor method.
Understanding classes and objects, along with their attributes and methods, is critical to mastering object-oriented programming. They form the basis for creating more complex and powerful programs.
In the next chapter, we'll explore more about object-oriented programming, including inheritance, polymorphism, and encapsulation.
Now answer the exercise about the content:
_Which of the following statements is true about object-oriented programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
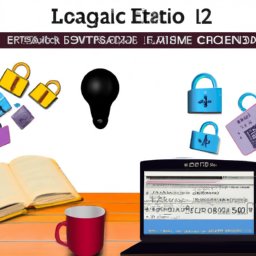
Next page of the Free Ebook: