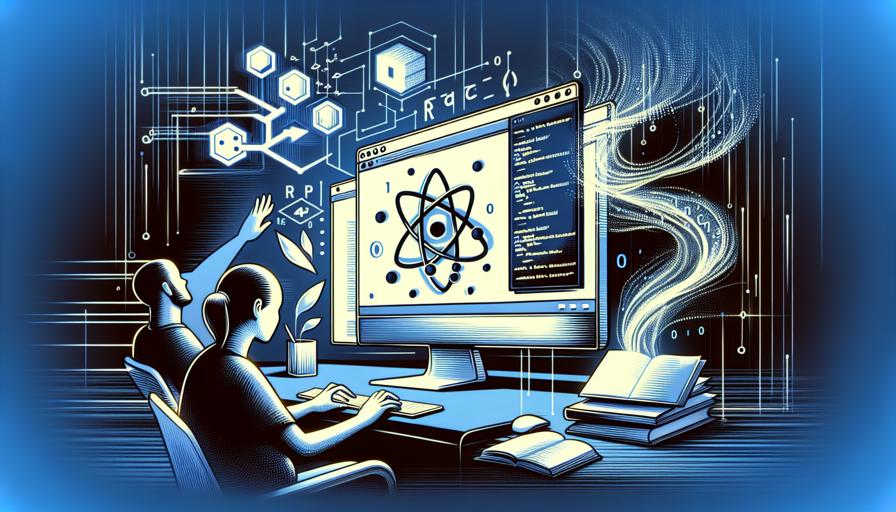
57. Building a Blogging Platform with React
Page 101 | Listen in audio
Building a blogging platform with React is an excellent way to solidify your understanding of React concepts while creating a practical and potentially scalable application. This project will cover various aspects of React, including component architecture, state management, routing, and integration with external APIs. By the end of this journey, you'll have a functional blogging platform that allows users to read, create, update, and delete blog posts.
Setting Up Your Environment
Before diving into the development of your blogging platform, ensure you have Node.js and npm (Node Package Manager) installed on your machine. These tools are essential for setting up a React environment. Once you have them installed, use the Create React App to bootstrap your project:
npx create-react-app blogging-platform
Navigate into your project directory:
cd blogging-platform
Start your development server to ensure everything is set up correctly:
npm start
Your default web browser should open a new tab displaying the React logo, indicating that your development environment is ready.
Structuring Your Application
React applications are structured using components. For a blogging platform, you might consider the following component hierarchy:
- App: The root component.
- Header: Displays the navigation bar.
- Footer: Displays footer information.
- BlogList: Displays a list of blog posts.
- BlogPost: Displays individual blog post details.
- CreatePost: Form for creating a new post.
- EditPost: Form for editing an existing post.
Begin by creating a components
directory inside src
and create separate files for each component. For instance:
src/
components/
Header.js
Footer.js
BlogList.js
BlogPost.js
CreatePost.js
EditPost.js
Implementing Routing
React Router is a popular library for handling routing in React applications. Install it using npm:
npm install react-router-dom
In your App.js
, import the necessary components from React Router:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
Set up your routes to render different components based on the URL:
<Router>
<Header />
<Switch>
<Route path="/" exact component={BlogList} />
<Route path="/post/:id" component={BlogPost} />
<Route path="/create" component={CreatePost} />
<Route path="/edit/:id" component={EditPost} />
</Switch>
<Footer />
</Router>
This setup will allow navigation between the home page (list of blogs), individual blog posts, and forms for creating or editing posts.
Fetching and Displaying Blog Posts
For a functional blogging platform, you need a way to store and retrieve blog posts. While a backend service is ideal for production, you can simulate this with a JSON file or an external API like JSONPlaceholder for development purposes.
Assuming you use JSONPlaceholder, fetch data in your BlogList
component:
import React, { useState, useEffect } from 'react';
function BlogList() {
const [posts, setPosts] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => setPosts(data));
}, []);
return (
<div>
{posts.map(post => (
<div key={post.id}>
<h2>{post.title}</h2>
<p>{post.body}</p>
</div>
))}
</div>
);
}
export default BlogList;
This component fetches posts from the API and displays them. Each post is rendered with a title and body.
Creating and Editing Posts
For creating and editing posts, you'll need forms to capture user input. Use controlled components in React to manage form data.
CreatePost Component
Set up a form in the CreatePost
component:
import React, { useState } from 'react';
function CreatePost() {
const [title, setTitle] = useState('');
const [body, setBody] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
const newPost = { title, body };
// Logic to send newPost to server
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={title}
onChange={e => setTitle(e.target.value)}
placeholder="Title"
required
/>
<textarea
value={body}
onChange={e => setBody(e.target.value)}
placeholder="Body"
required
/>
<button type="submit">Create Post</button>
</form>
);
}
export default CreatePost;
This form captures the title and body of a new post. On submission, you would typically send this data to a backend server to create a new post.
EditPost Component
The EditPost
component will be similar, but it will pre-fill the form with the existing post data. You can fetch the post data using the post ID from the URL parameters.
import React, { useState, useEffect } from 'react';
import { useParams } from 'react-router-dom';
function EditPost() {
const { id } = useParams();
const [title, setTitle] = useState('');
const [body, setBody] = useState('');
useEffect(() => {
fetch(`https://jsonplaceholder.typicode.com/posts/${id}`)
.then(response => response.json())
.then(data => {
setTitle(data.title);
setBody(data.body);
});
}, [id]);
const handleSubmit = (e) => {
e.preventDefault();
const updatedPost = { title, body };
// Logic to update the post on the server
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={title}
onChange={e => setTitle(e.target.value)}
placeholder="Title"
required
/>
<textarea
value={body}
onChange={e => setBody(e.target.value)}
placeholder="Body"
required
/>
<button type="submit">Update Post</button>
</form>
);
}
export default EditPost;
This component allows users to edit an existing post. It fetches the current post data and populates the form fields accordingly.
State Management and Optimization
As your application grows, managing state effectively becomes crucial. For this project, React's built-in useState
and useEffect
hooks are sufficient. However, for larger applications, consider using a state management library like Redux or Context API.
Additionally, optimize your application by implementing lazy loading for components, memoization with React.memo
, and using useCallback
and useMemo
hooks where applicable.
Styling Your Application
Styling is an essential aspect of any application. You can use CSS, CSS-in-JS libraries like styled-components, or frameworks like Bootstrap or Material-UI for styling your blogging platform.
For instance, using styled-components, you can create styled components for your form elements:
import styled from 'styled-components';
const Form = styled.form`
display: flex;
flex-direction: column;
max-width: 600px;
margin: auto;
`;
const Input = styled.input`
margin-bottom: 1rem;
padding: 0.5rem;
font-size: 1rem;
`;
const Textarea = styled.textarea`
margin-bottom: 1rem;
padding: 0.5rem;
font-size: 1rem;
`;
const Button = styled.button`
padding: 0.5rem;
font-size: 1rem;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
`;
function CreatePost() {
// ... component logic
return (
<Form onSubmit={handleSubmit}>
<Input
type="text"
value={title}
onChange={e => setTitle(e.target.value)}
placeholder="Title"
required
/>
<Textarea
value={body}
onChange={e => setBody(e.target.value)}
placeholder="Body"
required
/>
<Button type="submit">Create Post</Button>
</Form>
);
}
Styling enhances user experience and makes your application visually appealing.
Deploying Your Blogging Platform
Once your blogging platform is complete, consider deploying it to a hosting service like Vercel, Netlify, or GitHub Pages. These platforms offer straightforward deployment processes for React applications.
For instance, to deploy with Vercel, you can use the following steps:
- Install Vercel CLI:
npm install -g vercel
- Run
vercel
in your project directory. - Follow the prompts to deploy your application.
Vercel will provide a URL where your application is live, allowing you to share your blogging platform with others.
Conclusion
Building a blogging platform with React is a rewarding project that covers essential React concepts and provides a foundation for more advanced development. By creating components, managing state, implementing routing, and styling your application, you've gained valuable experience that will serve you well in future projects. Continue exploring React's ecosystem and consider integrating additional features like user authentication, comments, and categories to enhance your platform further.
Now answer the exercise about the content:
What is the purpose of building a blogging platform with React, as described in the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
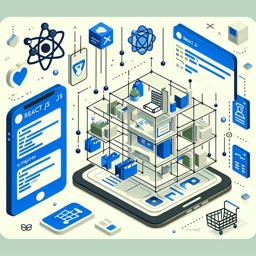
Next page of the Free Ebook: