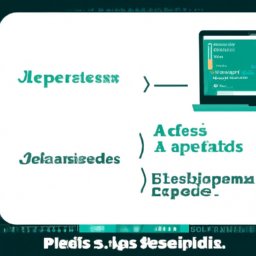
10.12. Building a Basic REST API with NodeJS and ExpressJS: Automated Tests
Page 77 | Listen in audio
Chapter 10.12 of our e-book course: "Building a Basic REST API with NodeJS and ExpressJS: Automated Testing". This chapter teaches you how to implement automated tests in your API using NodeJS and ExpressJS, an extremely popular library for creating APIs in NodeJS.
Automated testing is a crucial part of software development. They help ensure that your code is working correctly and that the changes you make don't break existing functionality. When developing an API, automated tests are even more important as they help ensure that the API is returning the correct data and correctly responding to multiple requests.
To start, let's install ExpressJS. If you already have NodeJS installed, you can install ExpressJS with the 'npm install express' command. After installation, you can create a new 'app.js' file and import ExpressJS with 'const express = require('express')'. Then you can create a new ExpressJS instance with 'const app = express()'.
With the ExpressJS instance created, you can start defining the routes for your API. For example, you can create a simple GET route that returns a JSON object with a welcome message. This can be done with the following code:
app.get('/', (req, res) => { res.json({ message: 'Welcome to our API!' }); });
With this route defined, you can start the ExpressJS server with 'app.listen(3000)', which will cause the server to start listening on port 3000. Now, if you access 'http://localhost: 3000' in your browser, you should see the welcome message.
Now that we have a basic API working, we can start implementing automated tests. For this, we are going to use a library called Jest. Jest is a popular testing library for JavaScript that offers a variety of features, including the ability to simulate HTTP requests.
You can install Jest with 'npm install --save-dev jest'. After installation, you can create a new 'app.test.js' file. In this file, we are going to import Jest and supertest, a library that allows us to simulate HTTP requests.
const request = require('supertest'); const app = require('./app');
With Jest and supertest imported, we can start writing our tests. Let's start with a simple test for our GET route. The test should send a GET request to the route and verify that the response is the JSON object we expect.
test('GET /', async() => { const response = await request(app).get('/'); expect(response.statusCode).toBe(200); expect(response.body).toEqual({ message: 'Welcome to our API!' }); });
This test uses supertest's 'request' function to send a GET request for the '/' route. It then checks that the response status code is 200, which indicates a successful response. It also checks that the response body is the JSON object we expect.
With this test in place, you can run 'npm test' to run the tests. If everything is set up correctly, you should see output indicating that the test passed.
Automated tests are a crucial part of API development, and with NodeJS and ExpressJS, implementing these tests is easy. However, this is just the beginning. There are many other aspects of testing that you can explore, including unit testing, integration testing, and load testing. But with the knowledge you've gained in this chapter, you're well equipped to get started.
We hope you found this chapter useful and informative. Stay tuned for the next chapter where we'll explore more advanced features of NodeJS and ExpressJS!
Now answer the exercise about the content:
What is the main purpose of automated testing in API development, as discussed in chapter 10.12 of the e-book course "Creating a Basic REST API with NodeJS and ExpressJS: Automated Testing"?
You are right! Congratulations, now go to the next page
You missed! Try again.
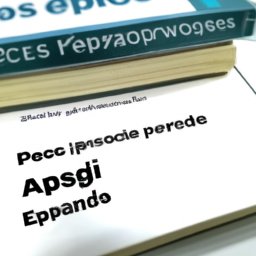
Next page of the Free Ebook: