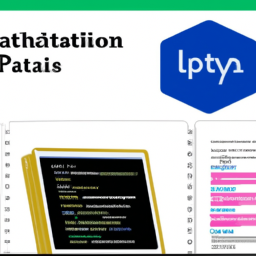
6.12. Object-Oriented Programming in Python: Interfaces in Python
Página 31
Object-oriented programming (OOP) is a programming paradigm that uses "objects" and their interactions to design applications and software programs. Within OOP, an interface is a way of defining the behavior that a class should have. In Python, we don't have the concept of "interface" like in other programming languages like Java or C#. However, we can create classes that function as an interface using abstract classes. Abstract classes in Python are used to define methods that must be implemented in subclasses.
For example, suppose we have an abstract class called "Animal". This class can define an abstract method called "talk". Any class that inherits from "Animal" must implement the "speak" method. So, if we have a "Dog" class and a "Cat" class that inherit from "Animal", both must implement the "talk" method.
```python from abc import ABC, abstractmethod class Animal(ABC): @abstractmethod def speak(self): pass class Dog(Animal): def speak(self): return 'Au Au' class Cat(Animal): def speak(self): return 'Meow' ```With this code, if we try to create an instance of the "Animal" class, we will receive an error as we cannot instantiate an abstract class. However, we can create instances of the "Dog" and "Cat" classes, and both will have the "talk" method.
```python dog = Dog() print(dog.talk()) # Au Au cat = Cat() print(cat.talk()) # Meow ```Interfaces in Python are important because they allow us to define a contract for classes. This contract states that any class that inherits from the abstract class must implement certain methods. This is useful because it allows us to write code that works with any class that implements a given interface, without worrying about the specific details of how that class implements the methods.
For example, we can have a function that accepts an object of type "Animal" and calls the "talk" method. This function will work with any class that inherits from "Animal", regardless of how the "talk" method is implemented.
```python def make_animal_talk(animal): print(animal.talk()) make_animal_talk(dog) # Au Au make_animal_talk(cat) # Meow ```In summary, interfaces in Python, implemented through abstract classes, allow us to define a contract that classes must follow. This allows us to write more generic and reusable code, as we can work with any object that implements a given interface, without worrying about the specific details of how that interface is implemented.
Now answer the exercise about the content:
What is the main function of abstract classes in the object-oriented programming paradigm in Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
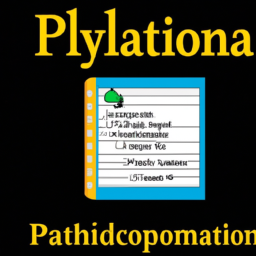
Next page of the Free Ebook: