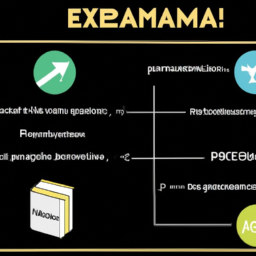
2.11. Python Language Fundamentals: Exception Handling in Python
Página 13
Exception handling is a crucial component of any programming language and Python is no exception. Learning how to handle exceptions in Python can help you avoid many common mistakes and make your code more robust and secure. This chapter of the course will cover the fundamentals of exception handling in Python, with a focus on how to use them effectively in backend development with Lambda and API Gateway.
In Python, exceptions are events that occur during program execution that interrupt the normal flow of the program. When an exception occurs, Python creates an exception object that contains information about the error that occurred. This exception object can then be caught and handled by a suitable block of code.
Exception handling in Python is performed using four keywords: try, except, finally and raise. The basic syntax of exception handling in Python is as follows:
try: # Code that can throw an exception exceptExceptionType: # Code that is executed if the exception is thrown finally: # Code that is always executed, regardless of whether an exception is thrown or not
The 'try' block contains code that can cause an exception. If an exception occurs in this block, the execution of the block is stopped and control is passed to the corresponding 'except' block. The 'except' block contains the code that is executed when an exception occurs. If no exception occurs in the 'try' block, the 'except' block is ignored. The 'finally' block, if present, is always executed, regardless of whether an exception was thrown or not.
Python supports several types of exceptions, including IOError, ValueError, ZeroDivisionError, ImportError, NameError, TypeError and many others. You can also define your own custom exceptions, inheriting from the Exception base class.
The 'raise' keyword can be used to explicitly throw an exception. For example, you may want to throw an exception if a certain condition is not met. Here is an example:
if x < 0: raise ValueError("x cannot be negative")
This code will throw a ValueError exception if x is less than zero.
Handling exceptions correctly is especially important when developing backend applications with Lambda and API Gateway. Lambda functions run in response to events, and it is crucial that they are able to handle all possible types of exceptions to avoid unexpected failures. Additionally, exceptions can be used to control the flow of program execution and provide meaningful responses to the client through API Gateway.
For example, you might want to throw a custom exception if a requested resource is not found, and then catch that exception to return an HTTP 404 response to the client. Here is an example of how this can be done:
try: # Code that searches for a resource except ResourceNotFoundException: # Returns an HTTP 404 response to the client via API Gateway finally: # Release any resources that have been allocated
In summary, exception handling is a fundamental part of the Python language that helps make code more robust and secure. When developing backend applications with Lambda and API Gateway, it is important to use exceptions effectively to control the flow of program execution and provide meaningful responses to the client.
Now answer the exercise about the content:
Which of the following statements about exception handling in Python is true?
You are right! Congratulations, now go to the next page
You missed! Try again.
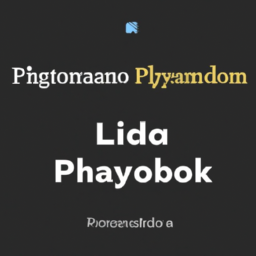
Next page of the Free Ebook: