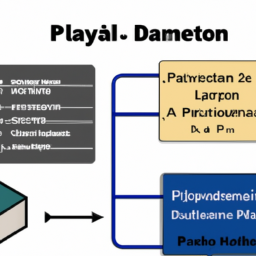
2.8. Python Language Fundamentals: Data Structures in Python
Página 10
2.8. Python Language Fundamentals: Data Structures in Python
Python is a high-level, interpreted, general-purpose programming language that stands out for its clear and readable syntax. One of the most important aspects of programming in Python is understanding the available data structures and how to use them effectively. In this section, we'll cover the fundamental data structures in Python: lists, tuples, sets, and dictionaries.
Lists
Lists in Python are a data structure that stores an ordered collection of items, which can be of any type. Lists are mutable, which means you can change their values. Lists are defined by placing items (elements) in square brackets [], separated by commas.
For example, you can create a list of integers as follows: numbers = [1, 2, 3, 4, 5]. Python also provides a number of methods that you can use to manipulate lists, such as append(), extend(), insert(), remove(), pop(), count(), sort(), reverse(), etc.
Tuples
Tuples are similar to lists in Python, but they are immutable, which means that we cannot change their elements after they are created. Tuples are used to store multiple items in a single variable. Tuples are defined by placing elements in parentheses (), separated by commas.
For example, you can create a tuple of colors as follows: colors = ('red', 'green', 'blue'). Just like lists, tuples also have methods, but not as many as lists, as tuples cannot be changed after creation.
Sets
Sets in Python are a collection of unordered and indexed items. Each element in a set is unique (not duplicated) and must be immutable (cannot be changed). However, a set itself is mutable. We can add or remove items from it. Sets are useful for performing mathematical operations like union, intersection, symmetric difference, etc.
For example, you can create a character set as follows: characters = {'a', 'b', 'c', 'd'}. Python provides a number of methods that you can use to manipulate sets, such as add(), update(), remove(), discard(), pop(), clear(), union(), intersection(), difference() , symmetric_difference(), etc.
Dictionaries
Dictionaries in Python are an unordered data structure that stores key-value pairs. Unlike other data structures that maintain only a single value as an element, dictionaries maintain a pair of values. The key, which is used to identify the item, and the value, which is the data we want to store. Dictionary keys must be unique and immutable.
For example, you can create a dictionary of students and their grades as follows: grades = {'John': 85, 'Maria': 90, 'Pedro': 78}. Python also provides a number of methods that you can use to manipulate dictionaries, such as keys(), values(), items(), get(), update(), pop(), popitem(), clear(), etc.
In summary, understanding and effectively using these data structures in Python can help you write more efficient and optimized code. Each data structure has its own strengths and weaknesses, and choosing the right data structure for a given problem can have a significant impact on the efficiency of your code.
Now answer the exercise about the content:
Which of the following statements about data structures in Python is true?
You are right! Congratulations, now go to the next page
You missed! Try again.
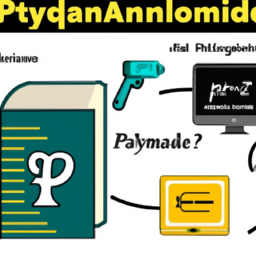
Next page of the Free Ebook: