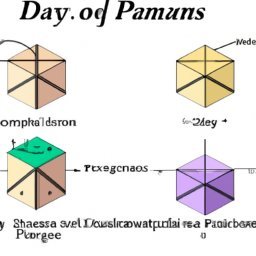
5. Data Structures in Python
Página 14
Data structures in Python
Python is a high-level, dynamic, interpreted, and easy-to-learn programming language. One of its most notable features is its ability to manipulate various data structures. In this chapter, we'll explore five fundamental data structures in Python: lists, tuples, sets, dictionaries, and strings.
Lists
The list is one of the most used data structures in Python. It is an ordered collection of items, which can be of any type. A list is defined by enclosing all items (elements) inside square brackets [], separated by commas.
list = [1, 2, 3, 4, 5]
Lists are mutable, which means that we can change an element of the list. In addition, lists support operations such as insertion, removal, and other operations that modify the list.
Tuples
Tuples are similar to lists, but they are immutable, which means that the elements of a tuple cannot be changed after they are assigned. Tuples are used to store multiple items in a single variable. They are defined by placing all items (elements) inside parentheses (), separated by commas.
tuple = (1, 2, 3, 4, 5)
Tuples are used when the order of the elements matters, or when the elements are immutable but the complete list is mutable.
Sets
A set is an unordered collection of unique items. Sets are used to eliminate duplicate items from a list and to perform mathematical operations such as union and intersection. Sets are defined by placing all items (elements) inside curly braces {}, separated by commas.
set = {1, 2, 3, 4, 5}
Sets are mutable and can be modified by adding or removing elements. However, sets do not support accessing, changing or removing elements using indexes due to the fact that they are unordered.
Dictionaries
A dictionary is an unordered collection of key-value pairs. It is generally used when we have a large dataset and we want to organize the data in a way that we can find it quickly. Dictionaries are defined by enclosing elements within braces {}, separated by commas. Each key-value pair is separated by a colon :
dictionary = {'name': 'John', 'age': 25, 'profession': 'Programmer'}
Dictionaries are mutable and we can add new key-value pairs or change the value of an existing key.
Strings
Strings in Python are sequences of characters. Python does not have a "char" data type, a single character is simply a string with a length of 1. Strings are defined by enclosing characters between single or double quotes.
string = "Hello world!"
Strings are immutable, which means that we cannot change a character in an existing string. However, we can concatenate strings or extract substrings from an existing string.
Understanding and mastering these data structures is critical to efficient programming in Python. Each data structure has its own characteristics and uses, and choosing the right data structure for a specific task can make your code more efficient and easier to understand.
Now answer the exercise about the content:
_Which of the following statements about data structures in Python is true?
You are right! Congratulations, now go to the next page
You missed! Try again.
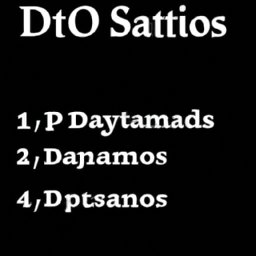
Next page of the Free Ebook: